C语言求二叉树中度为1的结点个数
时间: 2024-04-24 16:23:41 浏览: 15
要求二叉树中度为1的节点个数,可以通过遍历二叉树的方式来实现。以下是一个示例的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结点结构体
typedef struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建一个新的二叉树结点
TreeNode* createNode(int value) {
TreeNode* newNode = (TreeNode*)malloc(sizeof(TreeNode));
newNode->data = value;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
// 递归方式遍历二叉树,统计度为1的结点个数
int countNodesWithDegreeOne(TreeNode* root) {
if (root == NULL) {
return 0;
}
int count = 0;
// 当前结点的度为1,判断左子树和右子树是否为空
if ((root->left == NULL && root->right != NULL) || (root->left != NULL && root->right == NULL)) {
count++;
}
// 递归遍历左子树和右子树
count += countNodesWithDegreeOne(root->left);
count += countNodesWithDegreeOne(root->right);
return count;
}
int main() {
// 创建一个示例二叉树
TreeNode* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
// 统计度为1的结点个数
int count = countNodesWithDegreeOne(root);
printf("度为1的结点个数为:%d\n", count);
return 0;
}
```
在上面的示例代码中,我们使用了递归的方式遍历二叉树,并在遍历过程中统计度为1的结点个数。对于每个结点,我们检查其左子树和右子树是否为空,如果其中一个为空而另一个不为空,则该结点的度为1。最后返回统计的个数作为结果。在主函数中,我们创建了一个示例二叉树,并输出度为1的结点个数。
相关推荐
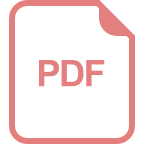
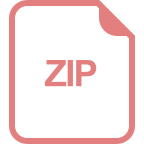














