用C语言实现:求给定二叉树的叶结点数、结点数、高度。
时间: 2024-05-02 22:22:35 浏览: 20
答:好的,我可以为您解答这个问题。
您可以参考以下 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 求给定二叉树的叶结点数
int leafNodeCount(TreeNode* root) {
if (!root) {
return 0;
}
if (!root->left && !root->right) {
return 1;
}
return leafNodeCount(root->left) + leafNodeCount(root->right);
}
// 求给定二叉树的结点数
int nodeCount(TreeNode* root) {
if (!root) {
return 0;
}
return 1 + nodeCount(root->left) + nodeCount(root->right);
}
// 求给定二叉树的高度
int height(TreeNode* root) {
if (!root) {
return 0;
}
int leftHeight = height(root->left);
int rightHeight = height(root->right);
return 1 + (leftHeight > rightHeight ? leftHeight : rightHeight);
}
int main() {
// 创建二叉树
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = NULL;
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->val = 3;
root->right->left = (TreeNode*)malloc(sizeof(TreeNode));
root->right->left->val = 4;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->right->val = 5;
root->right->right->left = NULL;
root->right->right->right = NULL;
// 求二叉树的叶结点数、结点数、高度
int leafCount = leafNodeCount(root);
int nodeCount = nodeCount(root);
int treeHeight = height(root);
printf("leafCount: %d, nodeCount: %d, treeHeight: %d\n", leafCount, nodeCount, treeHeight);
// 释放二叉树的内存
free(root->left);
free(root->right->left);
free(root->right->right);
free(root);
return 0;
}
```
上述代码创建了一个带有 5 个结点的二叉树,并且使用了三个函数来分别求二叉树的叶结点数、结点数和高度。您可以按照代码中的样式创建自己的二叉树,并调用这三个函数来获取所需的信息。
相关推荐
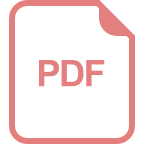
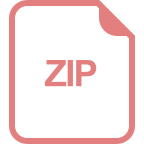














