A* 算法实现的伪代码,要求格式清晰,注释详细,解释每段伪代码的功能,并说明在实际编程实现中需要注意的细 节
时间: 2024-09-26 13:05:59 浏览: 33
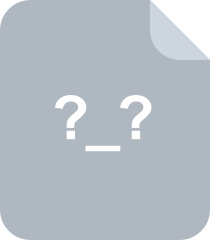
八数码问题求解:设计估价函数,给出算法伪代码,并采用c或python编程实现
A*算法是一种启发式搜索算法,用于寻找从起点到目标节点的最短路径。以下是A*算法的一个基本伪代码实现:
```text
// 定义节点类 Node
Node(Node parent, int g_cost, int h_cost, int f_cost) {
this.parent = parent; // 节点的前驱节点
this.g_cost = g_cost; // 从起始点到当前节点的实际代价
this.h_cost = h_cost; // 从当前节点到目标节点的估算代价
this.f_cost = g_cost + h_cost; // 全局估价函数,总成本
}
// 函数 start_search(start, goal)
List<Node> start_search(Node start, Node goal) {
PriorityQueue<Node> open_list = new PriorityQueue<>((a, b) => a.f_cost < b.f_cost); // 开放列表,优先级队列,按f_cost排序
open_list.add(start);
while (!open_list.isEmpty()) {
Node current = open_list.remove(); // 弹出开销最小的节点
if (current == goal) { // 如果找到目标节点,返回路径
return build_path(current);
}
// 探索当前节点的所有邻居
for (Node neighbor : expand(current)) {
int tentative_g_cost = current.g_cost + distance_between(current, neighbor); // 计算邻接节点的估计g值
// 如果邻居不在开放列表或新路径更优,则更新邻居状态
if (!open_list.contains(neighbor) || tentative_g_cost < neighbor.g_cost) {
neighbor.parent = current;
neighbor.g_cost = tentative_g_cost;
neighbor.h_cost = heuristic(neighbor, goal); // 更新h值
neighbor.f_cost = neighbor.g_cost + neighbor.h_cost;
// 将邻居放入开放列表并保持排序
open_list.add(neighbor);
}
}
}
// 没有找到路径,返回空列表
return null;
}
// 辅助函数 build_path(node)
List<Node> build_path(Node node) {
List<Node> path = new ArrayList<>();
while (node != null) {
path.add(node);
node = node.parent;
}
path.reverse(); // 逆序路径,从起点到终点
return path;
}
// 辅助函数 heuristic(node, goal)
int heuristic(Node node, Node goal) {
// 这里可以使用曼哈顿距离、欧几里得距离等估算方法,返回两点间直线距离
return ManhattanDistance(node.position, goal.position);
}
// 辅助函数 expand(node)
List<Node> expand(Node node) {
// 根据地图格特点,生成相邻节点列表
List<Node> neighbors = ...;
return neighbors;
}
```
注意事项:
1. 实际编程中,`distance_between()` 和 `heuristic()` 需要具体实现,通常根据场景选择合适的距离计算方法,如曼哈顿距离(棋盘格环境)、欧氏距离(连续空间)等。
2. 当创建`Node`实例时,`h_cost` 可能需要依赖于目标位置或地图特性来计算,例如,在二维网格上,可以用`(x2 - x1)^2 + (y2 - y1)^2`作为估算代价。
3. 为了提高效率,优化`expand()`函数以减少不必要的节点创建,比如只考虑可行的移动方向。
4. 在处理大规模数据时,要考虑内存管理和效率,可能会用到迭代加深搜索或广度优先搜索等变体。
阅读全文
相关推荐
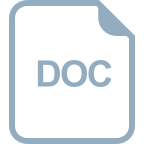
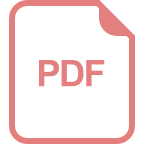















