假设线性表L=(al,a2,.,an)用顺序存储表示,试编写算法实现查找值为x的元素、若查找成功在其后插入值为k的元素。算法需包含以下三部分内容:存储结构定义,查找函数、插入函数。
时间: 2024-12-11 16:21:51 浏览: 10
在C语言中,我们可以使用结构体来定义线性表,然后创建相应的查找和插入函数。首先,我们定义一个`Node`结构体来表示列表中的每个节点,以及一个`List`结构体来表示整个线性表。
```c
// 存储结构定义
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
typedef struct List {
Node* head; // 链表头指针
int size; // 链表大小(用于记录元素数量)
} List;
```
接下来,我们需要编写两个函数:
1. 查找函数(`search_and_insert`),它接受一个值`x`和一个待查找位置`position`,如果找到`x`则返回该位置,否则返回-1。找不到时,在其后面插入值`k`。
```c
int search_and_insert(List* list, int x, int k, int position) {
int index = -1; // 初始化查找索引为-1
if (list->head == NULL) {
list->head = (Node*)malloc(sizeof(Node));
list->head->data = x;
list->size = 1;
return 0; // 如果链表为空直接插入第一个位置
}
Node* current = list->head;
for (int i = 0; i < list->size && index == -1; i++) {
if (current->data == x) {
index = i + 1; // 找到x的位置
}
current = current->next;
}
if (index != -1) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = k;
newNode->next = current;
list->size++;
if (position == index) { // 插入位置等于找到的位置
current = newNode;
} else if (position > index) { // 插入位置大于找到的位置
while (current->next != NULL && current->next->next != NULL) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
} else {
// 如果没找到x,则在末尾插入
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = k;
newNode->next = NULL;
list->head->next = newNode;
list->size++;
}
return index;
}
```
2. 插入函数(`insert_at_end`),如果要在一个特定位置插入新的元素,可以直接调用`search_and_insert`函数并将`position`设置为链表长度加上1。
```c
void insert_at_position(List* list, int k, int position) {
search_and_insert(list, -1, k, position);
}
```
现在你可以根据需要使用这些函数进行操作了。例如,创建一个新的链表并插入一个元素:
```c
List my_list = {NULL, 0};
insert_at_position(&my_list, 5, 0); // 在首位插入5
```
阅读全文
相关推荐
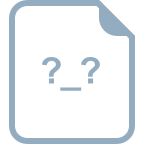
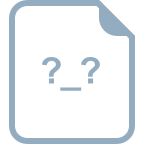
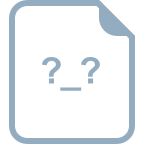

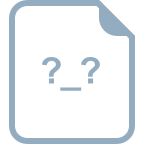













