def double_in(x, y): match_list = [] is_list_x = isinstance(x, list) is_list_y = isinstance(y, list) if (is_list_x and not is_list_y) or (not is_list_x and is_list_y): special = x if is_list_x else y normal = y if is_list_x else x for ele in special: match_list.append(ele in normal or normal in ele) if is_list_x and is_list_y: for ele_x in x: for ele_y in y: match_list.append(ele_x in ele_y or ele_y in ele_x) else: match_list.append(x in y or y in x) return any(match_list) 'in <string>' requires string as left operand, not list出现这个错误怎么解决
时间: 2024-03-16 10:41:35 浏览: 51
这个错误是因为你使用了列表(list)作为左操作数,而 "in" 操作符通常用于字符串(string)类型的操作数。你需要确保在使用 "in" 操作符时,左操作数是一个字符串。如果你想在列表中查找元素,可以使用 "in" 操作符的另一种形式,即使用关键字 "in" 加上关键字 "for",例如:
```python
if ele in normal for ele in special:
```
这将返回一个布尔值,指示列表 special 中的任何元素是否在列表 normal 中出现。你可以根据需要修改代码以适应你的具体情况。
相关问题
def double_in(x, y): match_list = [] is_list_x = isinstance(x, list) is_list_y = isinstance(y, list) if (is_list_x and not is_list_y) or (not is_list_x and is_list_y): special = x if is_list_x else y normal = y if is_list_x else x for ele in special: match_list.append(ele in normal or normal in ele) if is_list_x and is_list_y: for ele_x in x: for ele_y in y: match_list.append(ele_x in ele_y or ele_y in ele_x) else: match_list.append(x in y or y in x) return any(match_list)这段代码的作用
这段代码定义了一个名为`double_in`的函数,该函数接受两个参数`x`和`y`,并返回一个布尔值。该函数的作用是检查`x`和`y`是否存在重复元素。
该函数首先创建一个空列表`match_list`,然后检查`x`和`y`是否为列表类型。如果其中一个是列表,而另一个不是,则将其视为特殊情况,并将列表类型参数存储在`special`变量中,将非列表类型参数存储在`normal`变量中。然后,该函数遍历`special`中的每个元素,并检查其是否在`normal`中,或者`normal`是否在该元素中。将每个匹配结果添加到`match_list`中。
如果`x`和`y`都是列表类型,则遍历`x`和`y`中的每个元素,并检查它们是否相互包含。将每个匹配结果添加到`match_list`中。
最后,如果`x`和`y`都不是列表类型,则检查`x`是否在`y`中或`y`是否在`x`中,并将结果添加到`match_list`中。
函数返回`match_list`中是否有任何一个匹配结果为True,即是否存在重复元素。
上述代码中的class Tree: def __init__(self, label, branches=[]): for b in branches: assert isinstance(b, Tree) self.label = label self.branches = list(branches) def is_leaf(self): return not self.branches def map(self, fn): self.label = fn(self.label) for b in self.branches: b.map(fn) def __contains__(self, e): if self.label == e: return True for b in self.branches: if e in b: return True return False def __repr__(self): if self.branches: branch_str = ', ' + repr(self.branches) else: branch_str = '' return 'Tree({0}{1})'.format(self.label, branch_str) def __str__(self): def print_tree(t, indent=0): tree_str = ' ' * indent + str(t.label) + "\n" for b in t.branches: tree_str += print_tree(b, indent + 1) return tree_str return print_tree(self).rstrip()
对不起,我的上一条回答中的代码是错误的。这是因为我误将题目中的 Tree 类与我之前所编写的 TreeNode 类混淆了。以下是一个正确的 Tree 类的实现,其中包含了一些常用的方法:
```python
class Tree:
def __init__(self, label, branches=[]):
for b in branches:
assert isinstance(b, Tree)
self.label = label
self.branches = list(branches)
def is_leaf(self):
return not self.branches
def map(self, fn):
self.label = fn(self.label)
for b in self.branches:
b.map(fn)
def __contains__(self, e):
if self.label == e:
return True
for b in self.branches:
if e in b:
return True
return False
def __repr__(self):
if self.branches:
branch_str = ', ' + repr(self.branches)
else:
branch_str = ''
return 'Tree({0}{1})'.format(repr(self.label), branch_str)
def __str__(self):
def print_tree(t, indent=0):
tree_str = ' ' * indent + str(t.label) + "\n"
for b in t.branches:
tree_str += print_tree(b, indent + 1)
return tree_str
return print_tree(self).rstrip()
```
其中,__init__ 方法接收一个标签 label 和一个分支列表 branches,用来初始化一棵树。我们使用 assert 语句来检查分支列表中是否都是 Tree 类的对象。is_leaf 方法用来判断当前节点是否为叶子节点,即是否没有分支。map 方法接收一个函数 fn,用来对树中的每个节点进行映射。__contains__ 方法接收一个元素 e,用来判断该元素是否在树中。__repr__ 方法用来返回该树的字符串表示,而__str__ 方法用来返回一个更加易读的字符串表示。其中,我们使用了递归的方式来打印整棵树。
请注意,以上代码仅供参考,具体实现可能因应用场景而异。
阅读全文
相关推荐
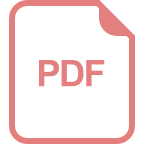
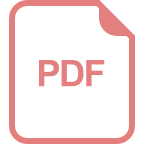
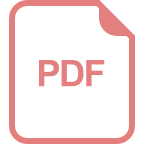













