G4Scintillation* scint = dynamic_cast<G4Scintillation*>(creator);
时间: 2024-05-27 19:08:19 浏览: 158
这段代码是 C++ 中的类型转换操作符 `dynamic_cast`,用于将 `creator` 指针所指向的对象转换为 `G4Scintillation` 类型的指针。
在这里,`creator` 可能是一个基类的指针,而 `G4Scintillation` 是这个基类的一个派生类。使用 `dynamic_cast` 进行转换可以确保转换是安全的,并且在转换失败时返回空指针。
如果转换成功,`scint` 将会指向一个 `G4Scintillation` 类型的对象,可以通过该指针调用 `G4Scintillation` 类中定义的成员函数和变量。如果转换失败,`scint` 将会是一个空指针,即 `nullptr`。
相关问题
G4Scintillation* scint = dynamic_cast<G4Scintillation*>(creator);dynamic_cast 无法丢掉常量或其他类型限定符,如何修改
如果在 dynamic_cast 中使用 const 限定符,你需要在转换目标指针类型时也加上 const 限定符。例如,如果 creator 是一个 const 指针,则可以将其转换为 const G4Scintillation* 类型的指针,如下所示:
```
const G4Scintillation* scint = dynamic_cast<const G4Scintillation*>(creator);
```
如果 creator 不是 const 指针,你可以将其转换为 G4Scintillation* 类型的指针,并在需要时使用 const_cast 操作符来去掉 const 限定符,如下所示:
```
G4Scintillation* scint = dynamic_cast<G4Scintillation*>(const_cast<G4VPhysicsConstructor*>(creator));
```
但是,应该谨慎使用 const_cast 操作符,因为它可能导致未定义的行为。如果你使用 const_cast 操作符去掉 const 限定符,而实际上对象是常量,那么修改将会导致未定义的行为。
如何使用G4Scintillation
要使用G4Scintillation包,需要在Geant4的代码中添加相应的头文件和初始化代码,并在代码中定义闪烁体材料的光学属性。以下是一个简单的示例代码,演示了如何使用G4Scintillation模拟闪烁体的荧光过程:
```
// 定义闪烁体材料
G4Material* scintillator = new G4Material("Scintillator", 1.032*g/cm3, 2);
scintillator->AddElement(G4Element::GetElement("C"), 9);
scintillator->AddElement(G4Element::GetElement("H"), 10);
// 定义闪烁体光学属性
G4MaterialPropertiesTable* scintillatorMPT = new G4MaterialPropertiesTable();
scintillatorMPT->AddConstProperty("SCINTILLATIONYIELD", 1000./MeV);
scintillatorMPT->AddConstProperty("RESOLUTIONSCALE", 1.0);
scintillatorMPT->AddConstProperty("FASTTIMECONSTANT", 1.*ns);
scintillatorMPT->AddConstProperty("SLOWTIMECONSTANT", 10.*ns);
scintillatorMPT->AddConstProperty("YIELDRATIO", 1.0);
scintillatorMPT->AddProperty("RINDEX", photonEnergy, rIndex, nEntries);
scintillatorMPT->AddProperty("ABSLENGTH", photonEnergy, absorption, nEntries);
scintillatorMPT->AddProperty("FASTCOMPONENT", photonEnergy, scintilFast, nEntries);
scintillatorMPT->AddProperty("SLOWCOMPONENT", photonEnergy, scintilSlow, nEntries);
scintillatorMPT->AddConstProperty("WLSTIMECONSTANT", 12.*ns);
scintillator->SetMaterialPropertiesTable(scintillatorMPT);
// 初始化闪烁体
G4Box* scintillatorSolid = new G4Box("ScintillatorSolid", 10.*mm, 10.*mm, 1.*mm);
G4LogicalVolume* scintillatorLogical = new G4LogicalVolume(scintillatorSolid, scintillator, "ScintillatorLogical");
G4VPhysicalVolume* scintillatorPhysical = new G4PVPlacement(0, G4ThreeVector(), scintillatorLogical, "ScintillatorPhysical", worldLogical, false, 0);
// 启用G4Scintillation
G4Scintillation* scintillationProcess = new G4Scintillation();
G4OpticalPhysics* opticalPhysics = new G4OpticalPhysics();
opticalPhysics->RegisterProcess(scintillationProcess, scintillator);
opticalPhysics->BuildPhysics();
```
在上述代码中,我们首先定义了一个名为“Scintillator”的闪烁体材料,然后设置了其光学属性。接着,我们创建了一个10x10x1mm的闪烁体几何体,并将其放置在世界几何体中。最后,我们创建了一个G4Scintillation对象,并使用G4OpticalPhysics将其注册到闪烁体材料中,以便在模拟中启用荧光过程。
需要注意的是,G4Scintillation包的使用需要对Geant4的代码有一定的了解,需要熟悉Geant4的几何体、材料、物理过程等相关概念。同时,在使用G4Scintillation包时,还需要根据具体应用场景调整相应的参数,以获得合适的模拟结果。
阅读全文
相关推荐
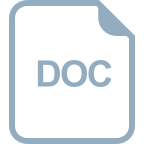
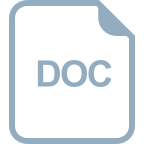
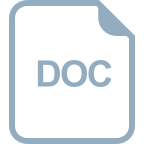
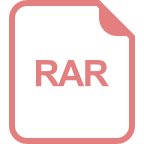
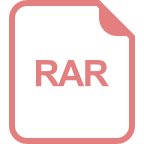











