用二叉树建立Tire树C++
时间: 2024-02-04 12:24:09 浏览: 110
你可以使用二叉树来实现Trie树(也称为字典树)。Trie树是一种用于高效存储和查找字符串的数据结构,它可以用于快速搜索和前缀匹配。
下面是一个使用二叉树实现Trie树的示例代码(C++):
```cpp
#include <iostream>
using namespace std;
const int ALPHABET_SIZE = 26;
// Trie节点
struct TrieNode {
TrieNode* children[ALPHABET_SIZE];
bool isEndOfWord;
};
// 初始化Trie节点
TrieNode* getNode() {
TrieNode* node = new TrieNode;
node->isEndOfWord = false;
for (int i = 0; i < ALPHABET_SIZE; i++) {
node->children[i] = nullptr;
}
return node;
}
// 插入字符串到Trie树
void insert(TrieNode* root, string key) {
TrieNode* curr = root;
for (int i = 0; i < key.length(); i++) {
int index = key[i] - 'a';
if (curr->children[index] == nullptr) {
curr->children[index] = getNode();
}
curr = curr->children[index];
}
curr->isEndOfWord = true;
}
// 查找字符串是否在Trie树中
bool search(TrieNode* root, string key) {
TrieNode* curr = root;
for (int i = 0; i < key.length(); i++) {
int index = key[i] - 'a';
if (curr->children[index] == nullptr) {
return false;
}
curr = curr->children[index];
}
return (curr != nullptr && curr->isEndOfWord);
}
int main() {
TrieNode* root = getNode();
insert(root, "apple");
insert(root, "banana");
insert(root, "orange");
cout << "Search 'apple': " << (search(root, "apple") ? "Found" : "Not Found") << endl;
cout << "Search 'banana': " << (search(root, "banana") ? "Found" : "Not Found") << endl;
cout << "Search 'orange': " << (search(root, "orange") ? "Found" : "Not Found") << endl;
cout << "Search 'grape': " << (search(root, "grape") ? "Found" : "Not Found") << endl;
return 0;
}
```
这段代码创建了一个Trie树,并演示了插入字符串和搜索字符串的过程。你可以根据需要修改和扩展它,以满足你的具体需求。
阅读全文
相关推荐
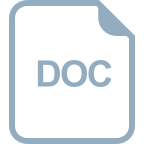
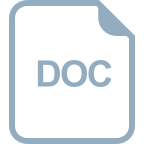
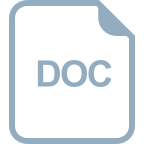
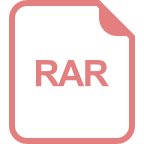
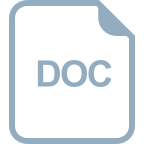
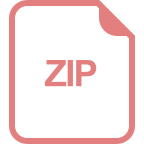
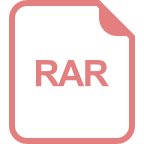
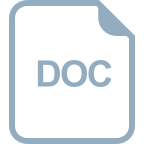
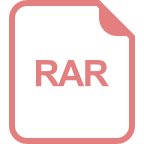
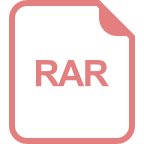
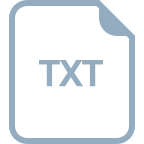
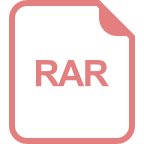