用二叉树建立字典树,用C++语言编写
时间: 2024-04-08 08:35:43 浏览: 73
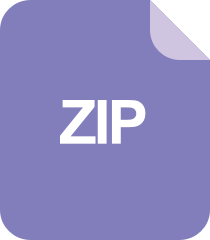
纯C++简易结构库,包括栈、队列、字典、排序、树的操作
可以使用二叉树来实现字典树,以下是用C++语言编写的一个示例代码:
```cpp
#include <iostream>
using namespace std;
const int ALPHABET_SIZE = 26;
struct TrieNode {
TrieNode* children[ALPHABET_SIZE];
bool isEndOfWord;
};
TrieNode* createNode() {
TrieNode* newNode = new TrieNode;
newNode->isEndOfWord = false;
for (int i = 0; i < ALPHABET_SIZE; i++) {
newNode->children[i] = NULL;
}
return newNode;
}
void insert(TrieNode* root, string word) {
TrieNode* cur = root;
for (int i = 0; i < word.length(); i++) {
int index = word[i] - 'a';
if (cur->children[index] == NULL) {
cur->children[index] = createNode();
}
cur = cur->children[index];
}
cur->isEndOfWord = true;
}
bool search(TrieNode* root, string word) {
TrieNode* cur = root;
for (int i = 0; i < word.length(); i++) {
int index = word[i] - 'a';
if (cur->children[index] == NULL) {
return false;
}
cur = cur->children[index];
}
return (cur != NULL && cur->isEndOfWord);
}
int main() {
TrieNode* root = createNode();
insert(root, "apple");
insert(root, "banana");
insert(root, "cherry");
cout << "Searching for 'apple': " << (search(root, "apple") ? "Found" : "Not Found") << endl;
cout << "Searching for 'banana': " << (search(root, "banana") ? "Found" : "Not Found") << endl;
cout << "Searching for 'cherry': " << (search(root, "cherry") ? "Found" : "Not Found") << endl;
cout << "Searching for 'grape': " << (search(root, "grape") ? "Found" : "Not Found") << endl;
return 0;
}
```
这段代码演示了如何使用二叉树实现字典树的插入和搜索操作。首先,我们创建了一个TrieNode结构体,其中包含一个布尔型变量`isEndOfWord`用于标记单词的结尾,以及一个包含26个子节点的数组`children`,用于存储每个字母的子节点。
然后,我们定义了一些辅助函数。`createNode`函数用于创建新的节点,`insert`函数用于将单词插入字典树,`search`函数用于搜索字典树中是否存在某个单词。
在主函数中,我们创建了一个空的字典树,并插入了几个单词。然后,我们通过调用`search`函数来搜索特定的单词,并输出搜索结果。
注意:这只是一个简单的示例代码,实际应用中可能需要考虑更多的功能和优化。
阅读全文
相关推荐
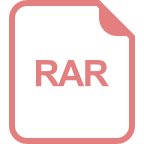
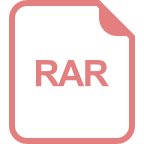















