解释函数TreeNode* buildTree(char* inorder, char* postorder, int inStart, int inEnd, int postStart, int postEnd) { // 递归终止条件 if (inStart > inEnd || postStart > postEnd) { return NULL; } // 创建根节点 TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode)); root->data = postorder[postEnd]; root->left = NULL; root->right = NULL; // 在中序遍历中找到根节点的位置 int rootIndex; for (rootIndex = inStart; rootIndex <= inEnd; rootIndex++) { if (inorder[rootIndex] == root->data) { break; } } // 构建左子树和右子树 root->left = buildTree(inorder, postorder, inStart, rootIndex - 1, postStart, postStart + (rootIndex - inStart) - 1); root->right = buildTree(inorder, postorder, rootIndex + 1, inEnd, postStart + (rootIndex - inStart), postEnd - 1); return root; }
时间: 2024-04-13 17:29:31 浏览: 34
这段代码是一个用于根据中序遍历和后序遍历序列构建二叉树的函数。函数定义如下:
```c
TreeNode* buildTree(char* inorder, char* postorder, int inStart, int inEnd, int postStart, int postEnd)
```
其中,`inorder`是中序遍历序列,`postorder`是后序遍历序列,`inStart`和`inEnd`表示中序遍历序列的起始和结束位置,`postStart`和`postEnd`表示后序遍历序列的起始和结束位置。
函数的实现逻辑如下:
1. 首先,通过判断递归终止条件来确定是否需要创建新的节点。如果中序遍历序列的起始位置大于结束位置,或者后序遍历序列的起始位置大于结束位置,则返回NULL。
2. 创建根节点,并将根节点的值设置为后序遍历序列的最后一个元素。
3. 在中序遍历序列中找到根节点的位置,通过遍历中序遍历序列,逐个比较元素与根节点的值,找到相等的位置。
4. 根据找到的根节点位置,将中序遍历和后序遍历序列划分为左子树和右子树的部分。左子树的范围为`inStart`到`rootIndex - 1`,`postStart`到`postStart + (rootIndex - inStart) - 1`;右子树的范围为`rootIndex + 1`到`inEnd`,`postStart + (rootIndex - inStart)`到`postEnd - 1`。
5. 递归调用`buildTree`函数构建左子树和右子树,并将返回的节点分别赋值给根节点的左子节点和右子节点。
6. 最后,返回根节点。
该函数的作用是根据给定的中序遍历和后序遍历序列构建出对应的二叉树。通过递归方式,不断划分序列范围,并创建节点,最终将所有节点连接起来,形成完整的二叉树结构。
希望对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
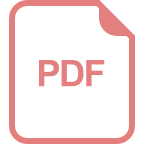
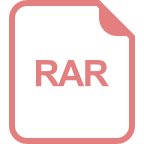
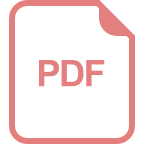














