C# 通过句柄获取截图
时间: 2024-10-07 09:01:49 浏览: 14
在C#中,如果你想要通过句柄(Handle)获取Windows桌面或特定窗口的截图,可以借助WinAPI函数结合GDI+(Graphics Device Interface)来实现。以下是一个基本步骤:
1. **获取句柄**:首先,你需要获取到你要截图窗口的句柄,这通常是通过`GetWindowThreadProcessId()`、`FindWindow()`或其他相关的Windows API函数。
2. **转换到设备上下文(DC)**:然后将句柄转换为Device Context(HDC),这对于屏幕捕捉至关重要。
3. **创建位图**:使用`CreateCompatibleBitmap()`创建一个与屏幕分辨率相同的位图。
4. **复制屏幕到位图**:使用`BitBlt()`或`CopyBits()`函数将屏幕复制到刚才创建的位图上。
5. **释放资源**:最后记得释放之前创建的所有GDI对象,包括位图和DC。
下面是一个简单的示例:
```csharp
using System.Runtime.InteropServices;
using System.Drawing;
// ...假设已经得到了窗口的句柄hwnd
[StructLayout(LayoutKind.Sequential)]
private struct RECT
{
public int Left;
public int Top;
public int Right;
public int Bottom;
}
[DllImport("user32.dll")]
static extern IntPtr GetDC(IntPtr hWnd);
[DllImport("gdi32.dll", SetLastError = true)]
static extern IntPtr CreateCompatibleBitmap(IntPtr hdc, int width, int height);
[DllImport("gdi32.dll")]
static extern bool BitBlt(IntPtr hdcDest, int xDest, int yDest, int width, int height, IntPtr hdcSrc, int xSrc, int ySrc, uint dwRop);
// ...
RECT rect = new RECT();
GetWindowRect(hwnd, out rect);
IntPtr hMemDC = GetDC(hwnd);
IntPtr hBitmap = CreateCompatibleBitmap(hMemDC, rect.Right - rect.Left, rect.Bottom - rect.Top);
BitBlt(hMemDC, 0, 0, rect.Width, rect.Height, hBitmap, 0, 0, Win32.BITMAP_SCOPY);
Bitmap bitmap = new Bitmap(rect.Width, rect.Height, hBitmap);
Graphics g = Graphics.FromImage(bitmap);
g.DrawImage(bitmap, 0, 0, bitmap.Width, bitmap.Height);
g.Dispose();
// 现在你可以对bitmap做进一步处理,比如保存图片或者显示
// 释放资源
DeleteObject(hBitmap);
ReleaseDC(hwnd, hMemDC);
```
相关推荐
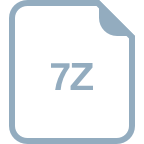
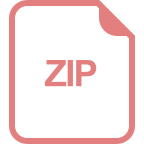
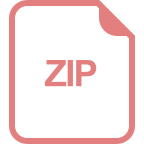














