MFC中怎么在一个旋转后的矩形内绘制椭圆
时间: 2024-03-20 14:45:35 浏览: 107
要在一个旋转后的矩形内绘制椭圆,可以使用GDI+中的Graphics对象实现。具体步骤如下:
1. 创建一个GDI+的Graphics对象,将其与MFC的DC对象关联。可以使用Gdiplus::Graphics的构造函数实现,例如:
```c++
Gdiplus::Graphics graphics(pDC->GetSafeHdc());
```
2. 在绘制椭圆之前,先将Graphics对象的坐标系旋转一个角度,可以使用Gdiplus::Graphics的RotateTransform方法。例如,如果要旋转30度,可以使用以下代码:
```c++
graphics.RotateTransform(30);
```
3. 创建一个矩形,可以使用Gdiplus::RectF结构体实现,例如:
```c++
Gdiplus::RectF rect(100, 100, 200, 150); // 创建一个矩形
```
4. 在矩形内绘制椭圆,可以使用Gdiplus::Graphics的DrawEllipse方法和Gdiplus::GraphicsPath类实现。具体步骤如下:
1. 创建一个Gdiplus::GraphicsPath对象,表示椭圆的路径,例如:
```c++
Gdiplus::GraphicsPath path;
```
2. 将椭圆的路径添加到GraphicsPath对象中,可以使用Gdiplus::GraphicsPath的AddEllipse方法实现,例如:
```c++
path.AddEllipse(rect);
```
3. 将GraphicsPath对象的路径裁剪为矩形的路径,可以使用Gdiplus::Graphics的SetClip方法实现,例如:
```c++
graphics.SetClip(rect);
```
4. 绘制椭圆,可以使用Gdiplus::Graphics的FillPath方法和Gdiplus::SolidBrush类实现,例如:
```c++
Gdiplus::SolidBrush brush(Gdiplus::Color(255, 0, 0)); // 创建一个红色的画刷
graphics.FillPath(&brush, &path); // 填充椭圆
```
5. 销毁Graphics对象,以便后续的绘制不受影响。可以使用delete运算符,例如:
```c++
delete graphics;
```
完整的绘制代码如下:
```c++
void CMyView::OnDraw(CDC* pDC)
{
// 创建一个GDI+的Graphics对象
Gdiplus::Graphics graphics(pDC->GetSafeHdc());
// 将Graphics对象的坐标系旋转一个角度
graphics.RotateTransform(30);
// 创建一个矩形
Gdiplus::RectF rect(100, 100, 200, 150);
// 创建一个GraphicsPath对象,表示椭圆的路径
Gdiplus::GraphicsPath path;
path.AddEllipse(rect);
// 将GraphicsPath对象的路径裁剪为矩形的路径
graphics.SetClip(rect);
// 绘制椭圆
Gdiplus::SolidBrush brush(Gdiplus::Color(255, 0, 0));
graphics.FillPath(&brush, &path);
// 销毁Graphics对象
delete graphics;
}
```
这样就可以在一个旋转后的矩形内绘制椭圆了。
阅读全文
相关推荐
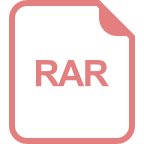
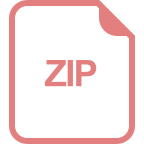
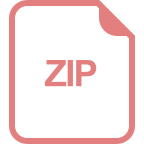










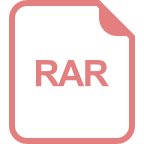
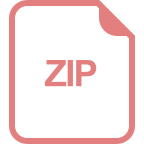
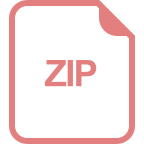
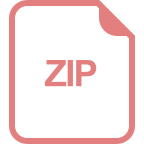
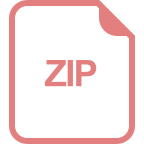
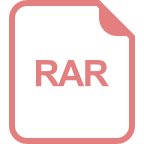
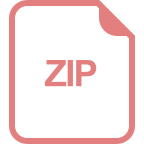