粒子滤波为什么要进行重采样
时间: 2024-01-05 14:04:21 浏览: 76
粒子滤波是一种基于蒙特卡洛方法的滤波算法,它通过在状态空间中产生一组粒子来近似概率分布。在每个时间步骤中,粒子根据其权重被重新采样,以使更多的粒子聚集在高概率区域,从而提高估计的准确性。
如果不进行重采样,那么在滤波过程中,一些粒子的权重会变得非常小,甚至接近于零。这些粒子对于表示概率分布的样本集合来说是没有意义的,因为它们不再代表概率分布的任何部分。另一方面,一些粒子的权重会变得非常大,这些粒子会在下一个时间步骤中占据所有样本的大部分,从而导致估计的方差变大。
通过重采样,可以保留高权重粒子并且减少低权重粒子的数量,从而保持样本的多样性并且提高估计的准确性。
相关问题
粒子滤波四种基本重采样代码
粒子滤波中的重采样是非常重要的一步,可以使得粒子在一定程度上避免漂移。以下是四种基本的重采样代码:
1. Systematic Resampling(系统重采样)
```python
def systematic_resampling(particles, weights):
N = len(weights)
positions = (np.arange(N) + np.random.uniform()) / N
indexes = np.zeros(N, 'i')
cumulative_sum = np.cumsum(weights)
i, j = 0, 0
while i < N and j < N:
if positions[i] < cumulative_sum[j]:
indexes[i] = j
i += 1
else:
j += 1
return particles[indexes]
```
2. Multinomial Resampling(多项式重采样)
```python
def multinomial_resampling(particles, weights):
indexes = np.zeros(len(weights), 'i')
cumulative_sum = np.cumsum(weights)
cumulative_sum[-1] = 1. # avoid round-off errors
for i in range(len(weights)):
indexes[i] = np.searchsorted(cumulative_sum, np.random.uniform())
return particles[indexes]
```
3. Residual Resampling(残余重采样)
```python
def residual_resampling(particles, weights):
N = len(weights)
indexes = np.zeros(N, 'i')
# determine integer parts for each weight
num_copies = (N * weights).astype(int)
k = 0
for i in range(N):
for _ in range(num_copies[i]):
indexes[k] = i
k += 1
# use multinomial resample on the residual to fill up the rest.
residual = weights - num_copies # get fractional part
residual /= sum(residual) # normalize
cumulative_sum = np.cumsum(residual)
cumulative_sum[-1] = 1. # avoid round-off errors
for i in range(k, N):
indexes[i] = np.searchsorted(cumulative_sum, np.random.uniform())
return particles[indexes]
```
4. Stratified Resampling(分层重采样)
```python
def stratified_resampling(particles, weights):
N = len(weights)
# make N subdivisions, and chose a random position within each one
positions = (np.random.uniform(0, 1, N) + range(N)) / N
indexes = np.zeros(N, 'i')
cumulative_sum = np.cumsum(weights)
i, j = 0, 0
while i < N and j < N:
if positions[i] < cumulative_sum[j]:
indexes[i] = j
i += 1
else:
j += 1
return particles[indexes]
```
粒子滤波四种基本重采样性能分析
粒子滤波(Particle Filter)是一种基于随机样本的滤波方法,用于解决非线性和非高斯问题。在粒子滤波算法中,通过一系列随机样本(粒子)来近似表示后验概率分布,从而实现状态估计和预测。
在粒子滤波中,重采样(Resampling)是一个重要的步骤,用于从当前的粒子集合中重新抽取一组新的粒子,以反映当前状态的不确定性。重采样有四种基本的方式,包括:
1. Multinomial Resampling: 多项式重采样,也称为轮盘赌重采样。该方法的思想是将一个区间[0,1]等分为N个子区间,每个子区间的长度对应粒子的权值,然后使用一个随机数生成器来选择新的粒子,从而实现重采样。
2. Residual Resampling: 剩余重采样。该方法在Multinomial Resampling的基础上进行了改进,通过先抽取整数部分的方式,将每个粒子的权值分成两部分,一部分用于产生新的粒子,另一部分则保留下来,以便在下一次重采样时使用。
3. Systematic Resampling: 系统重采样。该方法的思想是将整个粒子集合分成N个相同大小的子集,然后在每个子集中随机选择一个粒子作为重采样的代表。
4. Stratified Resampling: 分层重采样。该方法将每个粒子权值分成M个长度相等的子区间,然后在每个子区间中随机选取一个粒子作为新粒子。
以上四种重采样方法的性能分析如下:
1. Multinomial Resampling: 算法简单,计算速度快,但可能会出现粒子丢失或重复的问题。
2. Residual Resampling: 可以避免粒子丢失和重复的问题,但需要更多的计算量。
3. Systematic Resampling: 由于没有使用随机数生成器,因此可以避免出现粒子丢失和重复的问题,但会出现周期性的偏差。
4. Stratified Resampling: 可以避免粒子丢失和重复的问题,并且避免了Systematic Resampling的周期性偏差,但需要更多的计算量。
因此,在实际应用中,需要根据具体问题的要求选择最适合的重采样方法。
阅读全文
相关推荐
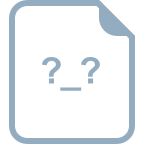
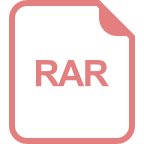
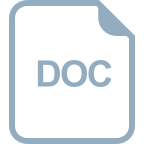
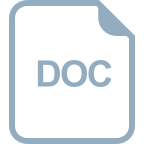







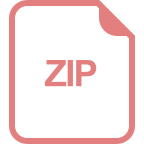
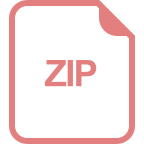