二叉树中值大于x的结点个数python,写出主程序
时间: 2024-02-18 11:01:09 浏览: 29
好的,以下是一个简单的二叉树实现和计算节点数量的Python主程序:
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
def count_nodes(root, x):
if root is None:
return 0
if root.value > x:
return 1 + count_nodes(root.left, x) + count_nodes(root.right, x)
else:
return count_nodes(root.right, x)
# 测试代码
if __name__ == "__main__":
root = None
values = [5, 3, 7, 1, 6, 8]
for v in values:
root = insert(root, v)
x = 4
count = count_nodes(root, x)
print(f"The number of nodes with value > {x} is {count}")
```
该程序首先定义了一个 `Node` 类表示二叉树节点,包括节点值、左右子节点。然后定义了 `insert` 函数用于向二叉树中插入节点。最后定义了 `count_nodes` 函数用于计算二叉树中值大于 `x` 的节点个数。
在主程序中,首先创建了一个空的根节点 `root`,然后向二叉树中插入一些节点。最后调用 `count_nodes` 函数计算二叉树中值大于 `x` 的节点个数,并输出结果。
请注意,这只是一个简单的二叉树实现和计算节点数量的示例,可能并不适用于所有情况。在实际开发中,可能需要根据具体需求进行修改和优化。
相关推荐
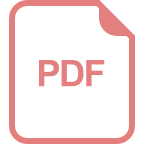














