Python代码假设二叉树中结点的类型为int,以二叉链表作为存储结构。求二叉树中值最大的结点。2. 假设二叉树中结点的类型为char,采用顺序存储。设计二叉树的先序、中序、后序和层次遍历算法。3. 在二叉链表上统计二叉树中度为2的结点个数
时间: 2024-12-19 13:16:04 浏览: 7
1. 如果二叉树节点的类型为整数(int),并使用二叉链表作为存储结构,要找到最大节点,可以采用递归或迭代的方式来遍历树。首先初始化一个当前最大值变量和一个指向根节点的指针,然后按照以下步骤操作:
- 如果当前节点为空,则返回已知的最大值。
- 比较当前节点的值和当前最大值,如果当前节点的值更大,更新最大值。
- 对左子节点和右子节点递归地执行同样的过程。
2. 当二叉树节点类型为字符(char)并采用顺序存储,遍历算法如下(这里仅提供伪代码):
- 先序遍历(根-左-右): 访问当前节点,然后遍历左子树,最后遍历右子树。
```python
def preorder_traversal(root):
if root is not None:
print(root.data)
preorder_traversal(root.left)
preorder_traversal(root.right)
```
- 中序遍历(左-根-右): 首先遍历左子树,然后访问当前节点,最后遍历右子树。
```python
def inorder_traversal(root):
if root is not None:
inorder_traversal(root.left)
print(root.data)
inorder_traversal(root.right)
```
- 后序遍历(左-右-根): 先遍历左右子树,最后访问当前节点。
```python
def postorder_traversal(root):
if root is not None:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.data)
```
- 层次遍历(广度优先搜索): 使用队列来存储每一层的节点。
```python
def level_order_traversal(root):
if root is None:
return
queue = [root]
while queue:
node = queue.pop(0)
print(node.data, end=" ")
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
```
3. 在二叉链表上统计度为2的节点个数,需要遍历每个节点并检查其左右子节点是否都存在。以下是Python代码示例:
```python
def count_degree_two_nodes(root):
count = 0
if root is not None:
if root.left and root.right:
count += 1
count += count_degree_two_nodes(root.left) + count_degree_two_nodes(root.right)
return count
```
阅读全文
相关推荐
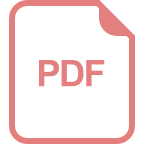
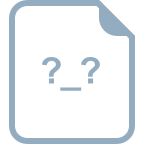
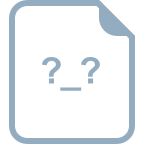















