brackets matching in a string
时间: 2024-02-15 15:53:40 浏览: 99
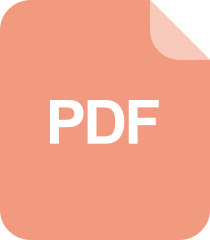
String Matching
Brackets matching in a string refers to the process of ensuring that all opening brackets have a corresponding closing bracket in the correct order. For example, the string "([]){}" is properly bracketed, while "([)]" is not, because the closing ")" does not match the opening "(".
There are several algorithms for checking whether a string has properly matched brackets. One common approach is to use a stack data structure, which allows you to keep track of the opening brackets as you encounter them and then check whether each closing bracket matches the most recent opening bracket. If all brackets match properly, then the stack will be empty at the end of the string.
阅读全文