MATLAB Reading of Cell Data in TXT Files: Structured Data Processing, Easily Handling Complex Data
发布时间: 2024-09-13 21:27:37 阅读量: 27 订阅数: 24 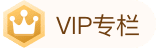
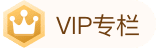
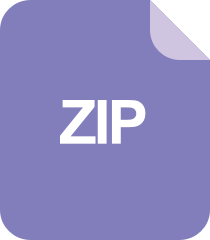
贝岭的matlab的代码-StructuredData_To_Descriptions:StructuredData_To_Descripti
# 1. MATLAB Reading of Cell Data in TXT Files: Structured Data Processing for Complex Data
MATLAB offers a variety of methods to read cell data from TXT files, including the use of the `textscan`, `importdata`, and `dlmread` functions.
- `textscan` Function: The `textscan` function uses regular expressions to parse text files and stores the data in a cell array. It provides flexible control, allowing users to specify data formats and delimiters.
- `importdata` Function: The `importdata` function is a more general function that can read various file formats, including TXT files. It can import data into cell arrays, matrices, or structures.
- `dlmread` Function: The `dlmread` function is specifically designed for reading delimited text files. It imports the data as a matrix but can convert it into a cell array.
# 2. MATLAB Cell Data Processing Tips
MATLAB cell data processing tips are powerful tools for effectively managing and operating cell arrays. This chapter will delve into the basic operations, conversions, and analyses of cell arrays, providing a comprehensive guide for users to handle cell data.
### 2.1 Basic Operations of Cell Arrays
#### 2.1.1 Creation and Initialization of Cell Arrays
There are two primary methods for creating cell arrays:
- **Using Braces ({}):** Cell elements are enclosed in braces, with each element separated by a comma. For example:
```matlab
myCellArray = {'Hello', 'World', 10, true};
```
- **Using the `cell()` Function:** The `cell()` function creates a cell array of specified size and type (optional). For example:
```matlab
myCellArray = cell(3, 2); % Creates a 3x2 cell array with elements of type object
```
#### 2.1.2 Indexing and Accessing Cell Arrays
Elements of a cell array can be accessed through indexing. The index can be a single number or a colon (:).
- **Single Index:** Accesses a specific cell. For example:
```matlab
element = myCellArray{2}; % Accesses the second element
```
- **Colon Index:** Accesses a subset of the cell array. For example:
```matlab
subArray = myCellArray{1:2}; % Accesses the first two elements
```
### 2.2 Conversions and Operations of Cell Arrays
#### 2.2.1 Conversions of Cell Arrays to Other Data Types
Cell arrays can be converted to other data types, such as strings, numbers, and structures.
- **Converting to Strings:** Use the `cellstr()` function to convert a cell array to a string array.
- **Converting to Numbers:** Use the `cell2mat()` function to convert a cell array to a numeric matrix.
- **Converting to Structures:** Use the `struct()` function to convert a cell array to a structure.
#### 2.2.2 Concatenation, Splitting, and Sorting of Cell Arrays
Cell arrays can be concatenated, split, and sorted to meet various processing needs.
- **Concatenation:** Use the [ ] operator to concatenate two or more cell arrays.
- **Splitting:** Use the `num2cell()` function to split numeric arrays into cell arrays.
- **Sorting:** Use the `sort()` function to sort the elements within a cell array.
### 2.3 Analysis and Processing of Cell Arrays
#### 2.3.1 Statistical Analysis of Cell Arrays
MATLAB provides functions for statistical analysis of cell arrays.
- **Length:** Use the `numel()` function to get the number of elements in a cell array.
- **Maximum and Minimum Values:** Use the `max()` and `min()` functions to find the maximum and minimum values within a cell array.
- **Mean and Median:** Use the `mean()` and `median()` functions to calculate the mean and median of a cell array.
#### 2.3.2 Text Processing of Cell Arrays
Text data within cell arrays can be processed using string manipulation functions.
- **Concatenation:** Use the `strcat()` function to concatenate strings within a cell array.
- **Finding and Replacing:** Use the `find()` and `strrep()` functions to find and replace text within a cell array.
- **Regular Expressions:** Use the `regexp()` function to perform regular expression matching on text within a cell array.
# 3 MATLAB Practical Applications of Cell Data
### 3.1 File Read and Write Operations with Cell Arrays
Cell arrays provide flexible file read and write functionality, making it convenient to handle text and binary files.
#### 3.1.1 Text File Read and Write with Cell Arrays
```matlab
% Create a cell array
data = {'MATLAB', 'is', 'a', 'high-level', 'programming', 'language.'};
% Write the cell array to a text file
fid = fopen('data.txt', 'w');
fprintf(fid, '%s\n', data{:});
fclose(fid);
% Read a cell array from a text file
fid = fopen('data.txt', 'r');
data = textscan(fid, '%s', 'Delimiter', '\n');
fclose(fid);
```
**Code Logic Analysis:**
* The `fopen` function opens a text file, with `'w'` indicating write mode.
* The `fprintf` function writes the elements of the cell array into the file, with `'%s\n'` specifying the format as strings followed by a newline.
* The `textscan` function reads data from the text file, with `'%s'` specifying the format as strings and `'Delimiter', '\n'` specifying the delimiter as the newline character.
#### 3.1.2 Binary File Read and Write with Cell Ar
0
0
相关推荐
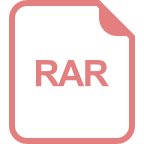
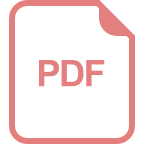
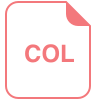
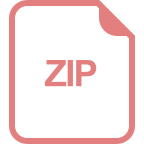
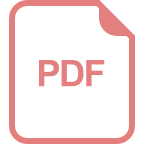
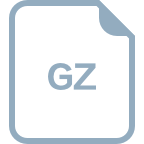
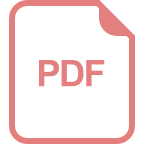
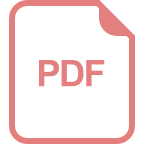