MATLAB Reading JSON Data from TXT Files: A Powerful Tool for Data Exchange, Easily Parsing JSON Format
发布时间: 2024-09-13 21:31:47 阅读量: 25 订阅数: 24 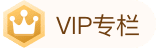
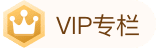
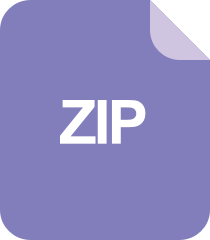
LazyJSON:一个非常快速,非常懒惰的Java JSON解析器
# An Introduction to Reading JSON Data in TXT Files Using MATLAB: A Powerful Tool for Data Exchange and Easy Parsing of JSON Formats
## 1. Introduction to JSON Data**
JSON (JavaScript Object Notation) is a lightweight data interchange format widely used in Web applications and APIs. It is based on the syntax of JavaScript objects, uses key-value pairs to store data, and is represented in a text format. JSON is easy to parse and generate, making it an ideal choice for cross-platform and language data transmission.
JSON data is typically represented as an object, where the keys are strings, and the values can be strings, numbers, booleans, arrays, or nested objects. Arrays represent an ordered set of values, and elements can be of any type of data.
## 2. Reading JSON Data in MATLAB
### 2.1 Introduction to the JSONlab Toolbox
JSONlab is an open-source toolbox for processing JSON data in MATLAB. It provides a wide range of functions for reading, parsing, converting, and writing JSON data. The JSONlab toolbox is developed and maintained by the MATLAB community and is available for free on the MATLAB Central File Exchange website.
### 2.2 Reading JSON Data with the JSONlab Toolbox
The JSONlab toolbox provides two primary functions for reading JSON data: `loadjson` and `jsondecode`.
#### 2.2.1 The `loadjson` Function
The `loadjson` function reads JSON data from a specified file path and loads it into a MATLAB structure. The syntax is as follows:
```
data = loadjson(filepath)
```
Where:
* `filepath`: The path to the text file containing the JSON data.
**Code Block:**
```
% Reading JSON data from a file
filepath = 'data.json';
data = loadjson(filepath);
```
**Logical Analysis:**
This code uses the `loadjson` function to read JSON data from the `data.json` file and load it into the `data` structure.
#### 2.2.2 The `jsondecode` Function
The `jsondecode` function decodes a JSON string into a MATLAB structure. The syntax is as follows:
```
data = jsondecode(jsonString)
```
Where:
* `jsonString`: A string containing JSON data.
**Code Block:**
```
% Reading JSON data from a string
jsonString = '{ "name": "John Doe", "age": 30 }';
data = jsondecode(jsonString);
```
**Logical Analysis:**
This code uses the `jsondecode` function to decode the JSON data in `jsonString` into the `data` structure.
### 2.3 Examples of Reading JSON Data
The following examples demonstrate how to use the JSONlab toolbox to read JSON data:
**Example 1: Reading JSON Data from a File**
```
% Reading JSON data from a file
filepath = 'data.json';
data = loadjson(filepath);
% Accessing JSON data
disp(data.name); % Outputs: "John Doe"
disp(data.age); % Outputs: 30
```
**Example 2: Reading JSON Data from a String**
```
% Reading JSON data from a string
jsonString = '{ "name": "Jane Doe", "age": 25 }';
data = jsondecode(jsonString);
% Accessing JSON data
disp(data.name); % Outputs: "Jane Doe"
disp(data.age); % Outputs: 25
```
## 3. Parsing JSON Data
### 3.1 Parsing JSON Data Structures
#### 3.1.1 Field Access
Fields in JSON data can be accessed using the dot operator `.`. For example, given the following JSON data:
```json
{
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main Street",
"city": "Anytown",
"state": "CA"
}
}
```
We can access the `name` field as follows:
```matlab
data = jsondecode(json_data);
name = data.name;
```
#### 3.1.2 Array Access
Arrays in JSON data can be accessed using square brackets `[]`. Array indexing starts at 0. For example, given the following JSON data:
```json
{
"names": ["John Doe", "Jan
```
0
0
相关推荐
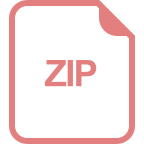
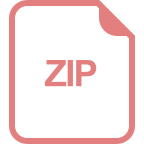
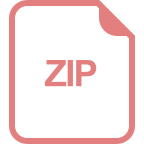
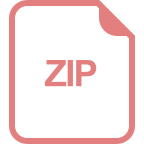
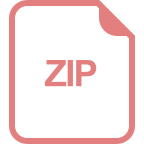
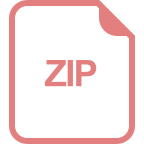
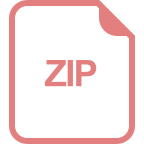
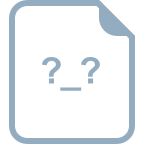