"MATLAB Masterclass: Reading TXT Files": Parsing Text Data to Enhance Data Processing Efficiency
发布时间: 2024-09-13 21:21:57 阅读量: 27 订阅数: 30 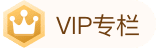
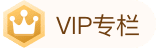

txt2xml: parsing text to xml-开源
# **MATLAB Reading TXT Files Guide**: Analyzing Text Data to Enhance Data Processing Efficiency
## 1. The Basics of MATLAB Reading TXT Files
Reading TXT files in MATLAB is a common operation for extracting data from text files. Text files store data in plain text format, usually ending with the `.txt` extension. MATLAB offers a variety of functions to read and process TXT files, including `fopen`, `fscanf`, and `textscan`.
The `fopen` function is used to open a TXT file and return a file identifier, which is used for subsequent read operations. The `fscanf` function reads data from the file according to a specified format and stores it in MATLAB variables. The `textscan` function is a more advanced function that allows users to customize data parsing rules and returns a cell array containing the parsed data.
## 2. Tips for Parsing Data from TXT Files
### 2.1 Data Type Identification and Conversion
#### Data Type Identification
By default, MATLAB treats all data read from a TXT file as strings. However, in practical applications, data may contain different data types, such as numbers, characters, dates, etc. It is necessary to identify the data types to process the data correctly.
MATLAB provides functions such as `isnumeric`, `ischar`, `islogical`, etc., to detect data types. For example:
```matlab
data = ['1', '2', '3', '4', '5'];
isnumeric(data) % false
ischar(data) % true
```
#### Data Type Conversion
After identifying the data type, it may need to be converted to another type for further processing. MATLAB offers various data type conversion functions, such as `str2num`, `num2str`, `logical`, etc.
For example, converting a string to a number:
```matlab
data = ['1', '2', '3', '4', '5'];
data_num = str2num(data); % [1, 2, 3, 4, 5]
```
### 2.2 Data Formatting and Processing
#### Data Formatting
Data in TXT files may have inconsistent formatting issues, such as numbers containing thousand separators or date formats being inconsistent. To facilitate processing, data needs to be formatted.
MATLAB provides functions like `strrep`, `regexprep` for string replacement and regular expression matching. For example, to remove thousand separators from numbers:
```matlab
data = ['1,000', '2,000', '3,000'];
data_formatted = strrep(data, ',', ''); % ['1000', '2000', '3000']
```
#### Data Processing
After data is formatted, it may require further processing, such as removing empty values, merging duplicates, etc. MATLAB provides functions like `isnan`, `unique` for data processing.
For example, removing empty values:
```matlab
data = ['1', '2', '', '4', '5'];
data_cleaned = data(~isnan(data)); % ['1', '2', '4', '5']
```
#### Code Block Example
```matlab
% Reading TXT file
data = importdata('data.txt');
% Identifying data types
isnumeric(data) % true
ischar(data) % false
% Converting data types
data_num = str2num(data);
% Formatting data
data_formatted = strrep(data_num, ',', '');
% Processing data
data_cleaned = data_formatted(~isnan(data_formatted));
```
#### Logical Analysis
The above code block is analyzed line by line as follows:
1. The `importdata` function reads the TXT file and returns the data.
2. The `isnumeric` function checks if the data is of numeric type.
3. The `str2num` function converts strings to numbers.
4. The `strrep` function removes thousand separators from numbers.
5. The `isnan` function checks if the data is NaN (Not a Number).
6. The `~isnan` function returns the indices of non-NaN elements.
## 3. Practical Applications of MATLAB Reading TXT Files
### 3.1 File Reading and Loading
**File Reading**
MATLAB offers several functions to read TXT files, with commonly used ones being:
```
fid = fopen('filename.txt', 'r');
data = textscan(fid, '%s %f %d');
fclose(fid);
```
* `fopen`:
0
0
相关推荐






