Reading Specific Columns and Rows from TXT Files in MATLAB: Fine-Grained Data Extraction to Meet Diverse Needs
发布时间: 2024-09-13 21:24:59 阅读量: 35 订阅数: 30 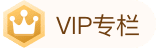
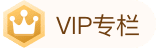
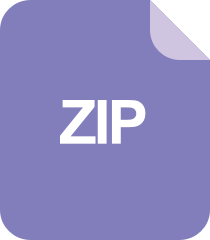
daany:Daany-.NET DAta AnalYtics .NET 5库,具有DataFrame,时间序列分解和线性代数例程BLASS和LAPACK的实现
# MATLAB Reading Specific Columns and Rows from TXT Files: Fine Data Extraction to Meet Diverse Needs
## 1. Basic MATLAB Reading of TXT Files
In MATLAB, reading text files (i.e., .txt files) can be accomplished using a variety of functions, depending on the format of the file and the type of data you wish to read. Here are some commonly used basic functions for reading text files and their descriptions:
### 1.1. `load`
- **Function**: Reads plain data text.
- **Usage**:
```matlab
T = readtable('filename.txt'); % For similar txt files without characters, only numbers
data = load('data_txt.txt');
x = data(:,1);
y = data(:,2);
plot(x,y,'r--');
```
### 1.2. `importdata`
- **Function**: If only the first row contains characters, then importdata can be used to directly read the data. The importdata function reads only the data, automatically skipping the characters before and after the data format.
- **Usage**:
```matlab
data1 = importdata('11.txt');
data2 = data1.data;
```
### 1.3. `textread`, `textscan`
- **Function**: Suitable for reading well-structured text, which will be stored in cells. The header lines (character rows) can be omitted using the headerlines option.
- **Usage**:
```matlab
[a1,a2,a3,a4] = textread('name.txt','%d%d%d%d','delimiter', ',','headerlines',1);
```
This code reads data from the `name.txt` file, skips the first row (usually the title), and stores the four integers from each row into variables `a1`, `a2`, `a3`, and `a4`. Each variable will contain all the data from the corresponding column.
### 1.4. `csvread`, `dlmread`
- **Function**: Suitable for reading text file formats such as csv, xsl, etc. Note: Starting from R2019a, `csvread` is recommended to be replaced by `readmatrix`.
- **Usage**:
```matlab
M = csvread('data_with_header.csv', 1, 0);
```
This code skips the title row and starts reading from the second row, used for reading CSV files with titles.
### 1.5. `fprintf`, `fscanf`
In MATLAB, `fprintf` and `fscanf` are functions used for file input and output, suitable for handling specific types of data. Below are their applicable scenarios and examples.
1. `fprintf`
Applicable Scenarios:
- **Writing formatted data to text files**: Suitable for writing numerical, string, etc., data in a specific format to a file.
- **Generating reports or log files**: Used for recording the results or status of program execution.
Example:
```matlab
% Open file to write
fid = fopen('output.txt', 'w');
% Write formatted data
fprintf(fid, 'The results are:\n');
fprintf(fid, 'Value 1: %.2f\n', 3.14159);
fprintf(fid, 'Value 2: %d\n', 42);
% Close the file
fclose(fid);
```
In this example, the content of `output.txt` will be:
```
The results are:
Value 1: 3.14
Value 2: 42
```
2. `fscanf`
Applicable Scenarios:
- **Reading formatted data from text files**: Suitable for reading structured numerical data, usually data that is separated by spaces or in a specific format.
- **Handling fixed-format data**: Suitable for reading files with a known format, such as experimental data or configuration files.
Example:
```matlab
% Open file to read
fid = fopen('data.txt', 'r');
% Read data from the file
data = fscanf(fid, '%f', [2, inf]); % Read floating-point numbers, stored by column
% Close the file
fclose(fid);
```
Assuming the content of `data.txt` is as follows:
```
*.***.*
*.***.0
5.0 6.0
```
In this example, `data` will be a 2x3 matrix:
```
data =
*.***.***.*
*.0 4.0 6.0
```
## 2. MATLAB Reading Specific Columns and Rows from TXT Files
### 2.1 Reading Specific Columns
Reading specific columns from a TXT file can help us extract the required data, avoiding unnecessary information processing. MATLAB provides various methods for reading specific columns, depending on the different delimiters.
#### 2.1.1 Reading Specific Columns Using Comma Delimiters
Comma delimiters are one of the most common delimiters for TXT files. To read specific columns using comma delimiters, the `textscan` function can be used:
```matlab
% Reading the file
data = textscan(fopen('data.txt'), '%s', 'Delimiter', ',');
% Extracting specific columns
specific_column = data{1}(:, 3);
```
**Code Logic Analysis:**
* The `textscan` function reads the file and stores the data in `data`.
* `%s` specifies reading string data.
* `Delimiter`, ',' specifies the comma as the delimiter.
* `data{1}` extracts the data, `(:, 3)` extracts the third column.
#### 2.1.2 Reading Specific Columns Using Space Delimiters
Space delimiters are also a common delimiter for TXT files. To read specific columns using space delimiters, the `strsplit` function can be used:
```matlab
% Reading the file
data = fileread('data.txt');
% Splitting the data
split_data = strsplit(data, ' ');
% Extracting specific columns
specific_column = split_data(:, 3);
```
**Code Logic Analysis:**
* The `fileread` function reads the file and stores the data in `data`.
* The `strsplit` function splits the data using spaces as the delimiter, stored in `split_data`.
* `(:, 3)` extracts the third column.
#### 2.1.3 Reading Specific Columns Using Custom Delimiters
If a TXT file uses a custom delimiter, the `regexp` function can be used to extract specific columns:
```matlab
% Reading the file
data = fileread('data.txt');
% Defining the delimiter
delimiter = '|';
% Splitting the data
split_data = regexp(data, delimiter, 'split');
% Extracting spec
```
0
0
相关推荐






