MATLAB Reading Character Data from TXT ***
发布时间: 2024-09-13 21:19:30 阅读量: 8 订阅数: 19 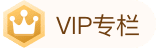
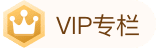
# 1. Overview of MATLAB Text File Reading
Reading text files in MATLAB is a fundamental task in data analysis and processing, allowing users to import data from text files and store it in MATLAB variables. Text files are often used to store structured or unstructured data, such as spreadsheets, log files, and text reports. MATLAB provides a variety of file reading functions that enable you to choose the most appropriate method based on your specific needs.
This chapter will cover the basics of MATLAB text file reading, including text file formats and encoding, an introduction to file reading functions, and common challenges in text file reading.
# 2. Basics of MATLAB Text File Reading
### 2.1 Text File Formats and Encoding
A text file is a computer file that stores plain text data, usually composed of one or more lines of text. Text files can come in various formats, such as:
- **CSV (Comma-Separated Values)**: Uses commas as field separators.
- **TSV (Tab-Separated Values)**: Uses tabs as field separators.
- **Fixed-Width Format**: Fields have a fixed width, padded with spaces or other characters.
- **JSON (JavaScript Object Notation)**: A lightweight dat***
***mon encodings include:
- **ASCII (American Standard Code for Information Interchange)**: A 7-bit encoding supporting 128 characters.
- **UTF-8 (8-bit Unicode Transformation Format)**: A variable-length encoding supporting over a million characters.
### 2.2 Introduction to File Reading Functions
MATLAB offers a variety of functions to read text files, the most commonly used of which include:
#### 2.2.1 textread
The `textread` function is used to read data from a text file and store it in a matrix. Its syntax is:
```
[data, count, msg] = textread(filename, formatSpec, sizeA, delimiter, headerLines, commentStyle)
```
Where:
- `filename`: The name of the text file.
- `formatSpec`: A string specifying the data format.
- `sizeA`: The size of the output matrix.
- `delimiter`: The field separator.
- `headerLines`: The number of lines to skip (usually header lines).
- `commentStyle`: The style of comment lines.
#### 2.2.2 fscanf
The `fscanf` function is used to read formatted data from a text file and store it in a vector or matrix. Its syntax is:
```
[data, count, msg] = fscanf(fid, formatSpec)
```
Where:
- `fid`: The file identifier.
- `formatSpec`: A string specifying the data format.
#### 2.2.3 textscan
The `textscan` function is used to read data from a text file and store it in a cell array. Its syntax is:
```
[data, delimiter, headerLines, commentStyle] = textscan(filename, formatSpec)
```
Where:
- `filename`: The name of the text file.
- `formatSpec`: A string specifying the data format.
- `delimiter`: The field separator.
- `headerLines`: The number of lines to skip (usually header lines).
- `commentStyle`: The style of comment lines.
**Code block:**
```
% Reading a CSV file
data = textread('data.csv', '%s %f %d', 'delimiter', ',');
% Reading a fixed-width format file
data = textread('data.txt', '%s %f %d', 'delimiter', ' ', 'whitespace', '');
% Reading a JSON file
data = jsondecode(fileread('data.json'));
```
**Logical Analysis:**
- The `textread` function uses `%s`, `%f`, and `%d` format specifiers to read string, floating-point, and integer data, respectively.
- The `fscanf` function uses `%s` and `%f` format specifiers to read string and floating-point data.
- The `textscan` function uses `%s` and `%f` format specifiers to read string and floating-point data, storing it in a cell array.
# 3. Tips for MATLAB Text File Reading
### 3.1 Data Type Conversion and Processing
#### 3.1.1 Numeric Data Conversion
MATLAB provides various functions for numeric data conversion, the most common of which are:
- `str2num`: Converts a string to a number.
- `str2double`: Converts a string to a double precision floating-point number.
- `str2int`: Converts a string to an integer.
**Code block:**
```matlab
% Converting a string to a number
num_str = '123.45';
num = str2num(num_str);
% Converting a string to a double precision floating-point number
double_num = str2double(num_str);
% Converting a string to an integer
int_num = str2int(num_str);
```
**Logical Analysis:**
* The `str2num` function converts the numeric part of a string to a number, ignoring spaces and commas.
* The `str2double` function converts the numeric part of a string to a double precision floating-point number, ignoring spaces and commas.
* The `str2int` function converts the numeric part of a string to an integer, ignoring spaces and commas.
#### 3.1.2 Character Data Processing
MATLAB provides various functions for character data processing, the most common of which are:
- `strtrim`: Removes spaces from the start and end of a string.
- `strrep`: Replaces specified characters or substrings within a string.
- `strsplit`: Splits a string into a cell array using a specified delimiter.
**Code block:**
```matlab
% Removing spaces from the start and end of a string
trimmed_str = strtrim(' Hello, world! ');
% Replacing specified characters or substrings within a string
replaced_str = strrep('This is a test', 'test', 'example');
% Splitting a string into a cell array using a specified delimiter
split_str = strsplit('This,is,a,test', ',');
```
**Logical Analysis:**
* The `strtrim` function removes spaces from the start and end of a string, returning the trimmed string.
* The `strrep` function replaces specified characters or substrings within a string, returning the replaced string.
* The `strsplit` function splits a string into a cell array using a specified delimiter, returning the cell array.
### 3.2 Handling Missing and Outlier Values
#### 3.2.1 Methods for Handling Missing Values
MATLAB provides various methods for handling missing values, the most common of which are:
- `isnan`: Determines if elements are NaN (Not a Number).
- `isinf`: Determines if elements are Inf (Infinity).
- `ismissing`: Determines if elements are missing values (NaN or Inf).
**Code block:**
```matlab
% Determining if elements are NaN
is_nan = isnan(data);
% Determining if elements are Inf
is_inf = isinf(data);
% Determining if elements are missing values
is_missing = ismissing(data);
```
**Logical Analysis:**
* The `isnan` function returns a logical array where NaN elements are true and all other elements are false.
* The `isinf` function returns a logical array where Inf elements are true and all other elements are false.
* The `ismissing` function returns a logical array where NaN and Inf elements are true and all other elements are false.
#### 3.2.2 Methods for Handling Outlier Values
MATLAB provides various methods for handling outlier values, the most common of which are:
- `isoutlier`: Determines if elements are outliers.
- `mad`: Calculates the Median Absolute Deviation (MAD), used to identify outliers.
- `iqr`: Calculates the Interquartile Range (IQR), used to identify outliers.
**Code block:**
```matlab
% Determining if elements are outliers
is_outlier = isoutlier(data);
% Calculating Median Absolute Deviation
mad_data = mad(data);
% Calculating Interquartile Range
iqr_data = iqr(data);
```
**Logical Analysis:**
* The `isoutlier` function returns a logical array where outlier elements are true and all other elements are false.
* The `mad` function calculates the Median Absolute Deviation, returning a scalar value.
* The `iqr` function calculates the Interquartile Range, returning a scalar value.
# 4. Practical Use of MATLAB Text File Reading
### 4.1 Data Import and Preprocessing
#### 4.1.1 Data Import
MATLAB provides multiple functions to import text files, including:
- `importdata`: Imports data from text files, supporting various formats.
- `textread`: Reads data from a text file and stores it in a matrix.
- `fscanf`: Reads formatted data from a text file.
- `textscan`: Reads data from a text file and stores it in a cell array.
The choice of function depends on the data format and processing needs. For example, if the data is comma-separated, the `textread` function can be used. If the data is in a fixed-width format, the `fscanf` function can be utilized.
```
% Importing a comma-separated text file
data = importdata('data.csv');
% Importing a fixed-width format text file
data = fscanf(fid, '%d %f %s', [3, inf]);
```
#### 4.1.2 Data Preprocessing
Before analyzing data, it is often necessary to preprocess the data to ensure consistency and completeness. Preprocessing steps may include:
- **Removing duplicates**: Using the `unique` function to remove duplicate rows or columns.
- **Handling missing values**: Using the `isnan` function to identify missing values and the `fillmissing` function to fill them in.
- **Converting data types**: Using the `str2num` function to convert strings to numbers, or the `num2str` function to convert numbers to strings.
- **Standardizing data**: Using the `zscore` function or the `normalize` function to standardize data, eliminating differences in scale.
```
% Removing duplicate rows
data = unique(data, 'rows');
% Filling in missing values
data = fillmissing(data, 'constant', 0);
% Converting strings to numbers
data(:, 1) = str2num(data(:, 1));
% Standardizing data
data = zscore(data);
```
### 4.2 Data Analysis and Visualization
#### 4.2.1 Data Analysis Methods
MATLAB provides multiple data analysis methods, including:
- **Statistical analysis**: Using functions like `mean`, `median`, `std` for statistical analysis.
- **Regression analysis**: Using the `fitlm` function for linear regression or the `fitglm` function for generalized linear models regression.
- **Cluster analysis**: Using the `kmeans` function or the `hierarchical` function for cluster analysis.
- **Principal component analysis**: Using the `pca` function for principal component analysis.
The choice of analysis method depends on the data type and research questions.
```
% Calculating mean and standard deviation
mean_data = mean(data);
std_data = std(data);
% Performing linear regression
model = fitlm(data(:, 1), data(:, 2));
% Performing cluster analysis
clusters = kmeans(data, 3);
```
#### 4.2.2 Data Visualization Methods
MATLAB provides multiple data visualization methods, including:
- **Scatter plot**: Using the `scatter` function to create a scatter plot.
- **Line plot**: Using the `plot` function to create a line plot.
- **Histogram**: Using the `histogram` function to create a histogram.
- **Box plot**: Using the `boxplot` function to create a box plot.
The choice of visualization method depends on the data type and the information to be conveyed.
```
% Creating a scatter plot
scatter(data(:, 1), data(:, 2));
% Creating a line plot
plot(data(:, 1), data(:, 2));
% Creating a histogram
histogram(data(:, 1));
% Creating a box plot
boxplot(data);
```
# 5. Advanced MATLAB Text File Reading
### 5.1 Large File Reading and Processing
#### 5.1.1 Large File Reading Optimization
When dealing with large text files, using default MATLAB file reading functions may lead to insufficient memory or low efficiency issues. To optimize large file reading, the following tips can be adopted:
- **Chunk reading**: Divide the large file into smaller chunks and read and process them one by one. This avoids loading the entire file into memory at once, thereby reducing memory consumption.
- **Streaming reading**: Use streaming reading functions, such as `textscan`, to read the file line by line. Streaming reading avoids loading the entire file into memory, thus improving efficiency.
- **Parallel reading**: If the file is large enough, parallel computing techniques can be used to distribute the file reading tasks to multiple processors, thereby increasing the reading speed.
#### 5.1.2 Large File Processing Tips
In addition to optimizing the reading process, when dealing with large text files, the following processing tips should be considered:
- **Data segmentation**: Divide the large file into smaller segments and process them one by one. This avoids processing the entire file at once, thereby reducing memory consumption and improving efficiency.
- **Data sampling**: For very large files, sampling techniques can be considered, processing only a portion of the file. This can save time and resources while still obtaining valuable information.
- **Data compression**: If the file contains a large amount of duplicate data, data compression techniques such as GZIP or BZIP2 can be used to reduce file size, thereby improving processing efficiency.
### 5.2 Text File Writing and Exporting
#### 5.2.1 Text File Writing
MATLAB provides various functions for writing data to text files, including:
- `fprintf`: Formats and writes to text files.
- `dlmwrite`: Writes to text files with delimiter-separated values.
- `csvwrite`: Writes to text files in comma-separated values (CSV) format.
The following code example demonstrates how to use the `fprintf` function to write to a text file:
```
% Open the file
fid = fopen('data.txt', 'w');
% Write data
fprintf(fid, '%d %s %.2f\n', 1, 'John', 3.14);
% Close the file
fclose(fid);
```
#### 5.2.2 Exporting Data as Text Files
MATLAB also provides functions to export data as text files, including:
- `exportdata`: Exports data to various text formats such as CSV, TSV, and HTML.
- `writetable`: Exports table data to text files.
The following code example demonstrates how to use the `exportdata` function to export data as a CSV file:
```
% Prepare data
data = [1, 'John', 3.14; 2, 'Mary', 4.56];
% Export data
exportdata(data, 'data.csv', 'Delimiter', ',');
```
# 6. Application Cases of MATLAB Text File Reading
### 6.1 Data Cleaning and Preprocessing
Data cleaning and preprocessing are crucial steps in the application of text file reading, aiming to improve data quality and reliability.
#### 6.***
***mon methods include:
- **Removing duplicate data**: Using the `unique` function or the `rmdup` function to remove duplicate rows or columns.
- **Handling missing values**: Using the `isnan` function to identify missing values and replace them with means, medians, or other statistics as needed.
- **Converting data types**: Using the `str2num` function to convert strings to numbers, or the `num2str` function to convert numbers to strings.
- **Standardizing data**: Transforming data into a consistent format, such as converting dates to a specific format or converting measurement units to standard units.
#### 6.1.2 Data Preprocessing Techniques
Data preprocessing techniques aim to enhance the operability of the data, including:
- **Feature selection**: Identifying and selecting features most relevant to the target task.
- **Feature scaling**: Scaling feature values to the same range to improve model performance.
- **Dimensionality reduction**: Using techniques such as Principal Component Analysis (PCA) or Singular Value Decomposition (SVD) to reduce the number of features.
- **Data sampling**: Extracting representative samples from large data sets to improve computational efficiency.
### 6.2 Text Mining and Natural Language Processing
Text mining and Natural Language Processing (NLP) techniques can be used to extract valuable information from text files.
#### ***
***mon techniques include:
- **Text tokenization**: Breaking text into words or phrases.
- **Term frequency counting**: Counting the occurrence of each word or phrase.
- **Text classification**: Assigning text to predefined categories.
- **Text clustering**: Grouping text into similar topics.
#### ***
***mon techniques include:
- **Part-of-speech tagging**: Identifying the part of speech of words, such as nouns, verbs, or adjectives.
- **Syntactic parsing**: Analyzing the grammatical structure of sentences.
- **Semantic analysis**: Understanding the meaning of text.
- **Machine translation**: Translating text from one language to another.
0
0