编写一个学生和教师数据输入和显示程序,要求: (1)学生数据有编号、姓名、班号和成绩。 (2)教师数据有编号、姓名、职称和部门。 (3)将编号、姓名的输入和显示设计成类person,作为学生数据操作类student和教师数据操作类teacher的基类。用完整的准确的c++语言表示
时间: 2024-06-10 11:04:23 浏览: 95
#include <stdio.h>
#include <stdlib.h>
// 定义person类,包含编号和姓名
typedef struct {
int id; // 编号
char name[20]; // 姓名
} person;
// 学生数据操作类,继承person类
typedef struct {
person p; // 继承person类
int classNum; // 班号
float score; // 成绩
} student;
// 教师数据操作类,继承person类
typedef struct {
person p; // 继承person类
char title[20]; // 职称
char department[20]; // 部门
} teacher;
// 定义函数,用于输入一个person对象的信息
void inputPersonInfo(person *p) {
printf("请输入编号:");
scanf("%d", &(p->id));
printf("请输入姓名:");
scanf("%s", p->name);
}
// 定义函数,用于输出一个person对象的信息
void outputPersonInfo(person *p) {
printf("编号:%d\t", p->id);
printf("姓名:%s\t", p->name);
}
// 定义函数,用于输入一个学生对象的信息
void inputStudentInfo(student *s) {
inputPersonInfo(&(s->p));
printf("请输入班号:");
scanf("%d", &(s->classNum));
printf("请输入成绩:");
scanf("%f", &(s->score));
}
// 定义函数,用于输出一个学生对象的信息
void outputStudentInfo(student *s) {
outputPersonInfo(&(s->p));
printf("班号:%d\t", s->classNum);
printf("成绩:%f\n", s->score);
}
// 定义函数,用于输入一个教师对象的信息
void inputTeacherInfo(teacher *t) {
inputPersonInfo(&(t->p));
printf("请输入职称:");
scanf("%s", t->title);
printf("请输入部门:");
scanf("%s", t->department);
}
// 定义函数,用于输出一个教师对象的信息
void outputTeacherInfo(teacher *t) {
outputPersonInfo(&(t->p));
printf("职称:%s\t", t->title);
printf("部门:%s\n", t->department);
}
int main() {
student s1;
teacher t1;
printf("***输入学生信息***\n");
inputStudentInfo(&s1);
printf("\n***输入教师信息***\n");
inputTeacherInfo(&t1);
printf("\n***输出学生信息***\n");
outputStudentInfo(&s1);
printf("\n***输出教师信息***\n");
outputTeacherInfo(&t1);
system("pause");
return 0;
}
阅读全文
相关推荐
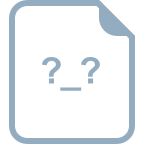
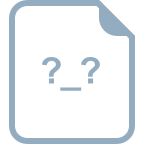











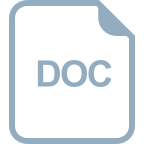
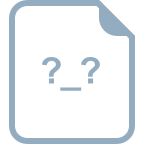