#include <stdio.h> #define N 4 // 定义学生结构体 struct student { long id; char name[10]; char gender; float score1; float score2; float score3; float total_score; float average_score; };// 计算学生总分和平均分的函数 void count(struct student *stu) { for (int i = 0; i < N; i++) { stu[i].total_score = stu[i].score1 + stu[i].score2 + stu[i].score3; stu[i].average_score = stu[i].total_score / 3.0; } } int main(void) { // 定义学生数组 struct student stu[N]; // 输入学生数据 printf("学号 姓名 性别 课1 课2 课3\n"); for (int i = 0; i < N; i++) { scanf("%ld%s %c%f%f%f", &stu[i].id, &stu[i].name, &stu[i].gender, &stu[i].score1, &stu[i].score2, &stu[i].score3); } // 计算每个学生的总分和平均分 count(stu); // 输出学生数据 printf("学号 姓名 性别 课1 课2 课3 总分 平均分\n"); for (int i = 0; i < N; i++) { printf("%8ld%10s%3c %8ld%10s%3c %8ld%10s%3c %6.1f %6.1f %6.1f\n", stu[i].id, stu[i].name, stu[i].gender, stu[i].score1, stu[i].score2, stu[i].score3, stu[i].total_score, stu[i].average_score); } return 0; }检查程序
时间: 2023-12-11 11:03:55 浏览: 76
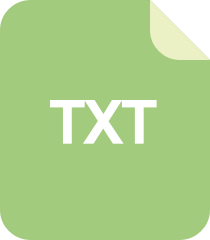
#include <stdio.h>

这是一段 C 语言程序,用于输入学生的学号、姓名、性别以及三门课的成绩,然后计算每个学生的总分和平均分,并输出所有学生的数据。程序中使用了一个结构体来表示每个学生的数据,并定义了一个计算总分和平均分的函数 count。
程序中的宏定义 #define N 4 定义了学生的数量,可以根据需要修改。在 main 函数中,首先定义了一个学生数组 stu[N],然后通过循环输入每个学生的数据。接着调用 count 函数计算每个学生的总分和平均分。最后,再次循环输出每个学生的数据,包括学号、姓名、性别、三门课的成绩、总分和平均分。
程序的语法看起来没有问题,但还需要测试和验证,确保程序的正确性和稳定性。
阅读全文
相关推荐
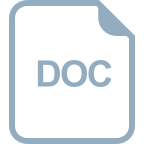

















