A*算法解决旅行商问题C++
时间: 2024-09-19 21:00:43 浏览: 60
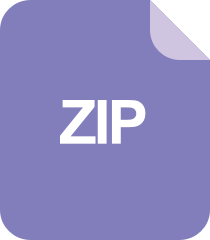
旅行商问题 A星算法求解
A*算法(A* Search Algorithm)是一种启发式搜索算法,常用于求解最短路径问题,包括旅行商问题(Traveling Salesman Problem, TSP)。旅行商问题是这样一个经典问题:给定一组城市及其之间的距离,找到一条路径,使得旅行商能够访问每个城市一次并返回起点,总行程最短。
在C++中实现A*算法解决TSP通常会涉及以下几个步骤:
1. **定义状态空间**:将每个城市的组合视为一个状态,并用一对坐标(当前城市位置,已访问的城市列表)表示。
2. **计算代价函数**:包括两部分,一是从上一步到当前状态的实际移动代价,通常是两个城市间的直接距离;二是估算从当前状态到目标状态的启发式估价,比如曼哈顿距离或欧几里得距离加上剩余未访问城市的最小距离。
3. **优先队列数据结构**:A*使用优先队列存储待处理的状态,按照F值(实际代价+启发式估计)排序,总是选择F值最小的节点进行扩展。
4. **开始迭代**:初始状态通常设置为所有城市的一次遍历,然后进入循环。每次从队列中取出F值最低的节点,如果它是目标状态,则找到解决方案;如果不是,将其相邻的未访问节点加入队列并更新它们的F值。
5. **剪枝策略**:为了减少搜索空间,A*算法只考虑那些看起来最有可能导致最优解的分支,通过不断调整启发式估价的精度来控制搜索深度。
6. **返回路径**:一旦找到解决方案,可以从最后一个添加进来的节点开始回溯,记录下经过的所有城市形成最终的旅行路线。
阅读全文
相关推荐
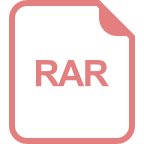
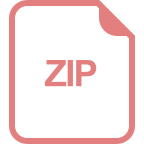
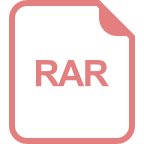
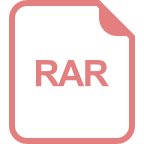
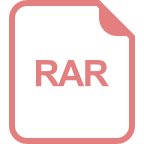

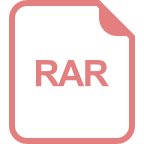
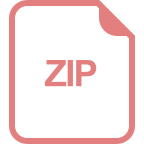
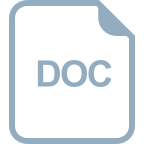
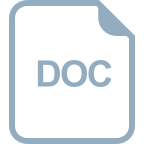
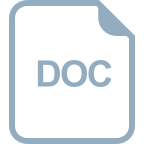






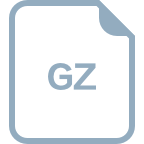
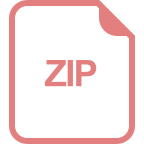