python 二维数组项城
时间: 2023-10-19 12:34:16 浏览: 156
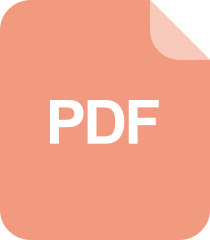
Python输入二维数组方法
要创建一个二维数组(矩阵)并对其进行操作,你可以使用 Python 的列表嵌套。以下是一个示例:
```python
# 创建一个3x3的二维数组
matrix = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# 访问二维数组的元素
print(matrix[0][0]) # 输出 1
print(matrix[1][2]) # 输出 6
# 修改二维数组的元素
matrix[2][1] = 10
print(matrix[2][1]) # 输出 10
# 遍历二维数组
for row in matrix:
for element in row:
print(element, end=" ")
print()
```
这将输出:
```
1 2 3
4 5 6
7 10 9
```
你可以根据需要修改数组大小、访问和修改元素,并在循环中遍历数组。
阅读全文
相关推荐
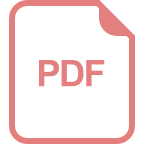
















