easy2d 写个俄罗斯方块 代码
时间: 2023-11-15 07:06:08 浏览: 59
好的,这是一个简单的俄罗斯方块游戏的代码示例:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
#include <conio.h>
#include <windows.h>
using namespace std;
const int ROW = 30;
const int COL = 20;
int score;
int map[ROW][COL];
struct Point {
int x, y;
Point(int x = 0, int y = 0) :x(x), y(y) {}
};
vector<Point> curShape;
vector<vector<Point>> shapes;
void initShapes() {
shapes.push_back({ {0,0}, {0,1}, {0,2}, {0,3} });
shapes.push_back({ {0,0}, {0,1}, {0,2}, {1,2} });
shapes.push_back({ {0,0}, {0,1}, {1,0}, {1,1} });
shapes.push_back({ {0,0}, {0,1}, {0,2}, {1,1} });
shapes.push_back({ {0,0}, {0,1}, {1,1}, {1,2} });
shapes.push_back({ {0,0}, {1,0}, {1,1}, {2,1} });
shapes.push_back({ {0,1}, {1,1}, {2,0}, {2,1} });
}
void newShape() {
curShape.clear();
srand((unsigned)time(NULL));
int index = rand() % shapes.size();
for (int i = 0; i < 4; i++) {
Point p = shapes[index][i];
curShape.push_back(Point(p.x + ROW / 2, p.y));
}
}
bool check(Point p) {
if (p.x < 0 || p.x >= ROW || p.y >= COL) return false;
if (map[p.x][p.y]) return false;
return true;
}
bool isOver() {
for (int i = 0; i < 4; i++) {
if (!check(curShape[i]))
return true;
}
return false;
}
void transform(Point& p) {
int tmp = p.x;
p.x = p.y;
p.y = 3 - tmp;
}
void rotate() {
Point center = curShape[1];
for (int i = 0; i < 4; i++) {
if (i == 1) continue;
int x = curShape[i].x - center.x;
int y = curShape[i].y - center.y;
Point t(x, y);
transform(t);
curShape[i].x = center.x + t.x;
curShape[i].y = center.y + t.y;
}
}
void updateMap() {
for (int i = 0; i < 4; i++) {
int x = curShape[i].x;
int y = curShape[i].y;
map[x][y] = 1;
}
}
bool full(int r) {
for (int c = 0; c < COL; c++) {
if (map[r][c] == 0) return false;
}
return true;
}
void removeRow(int r) {
for (int c = 0; c < COL; c++) {
map[r][c] = 0;
}
for (int i = r; i >= 1; i--) {
for (int c = 0; c < COL; c++) {
map[i][c] = map[i - 1][c];
}
}
for (int c = 0; c < COL; c++) {
map[0][c] = 0;
}
score++;
}
void checkFull() {
for (int r = ROW - 1; r >= 0; r--) {
if (full(r)) {
removeRow(r);
r++;
}
}
}
void refresh() {
system("cls");
cout << "score: " << score << endl;
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (map[i][j]) cout << "*";
else cout << " ";
}
cout << endl;
}
}
void moveLeft() {
for (int i = 0; i < 4; i++) {
Point p = curShape[i];
p.y--;
if (!check(p)) {
return;
}
}
for (int i = 0; i < 4; i++) {
curShape[i].y--;
}
}
void moveRight() {
for (int i = 0; i < 4; i++) {
Point p = curShape[i];
p.y++;
if (!check(p)) {
return;
}
}
for (int i = 0; i < 4; i++) {
curShape[i].y++;
}
}
void moveDown() {
if (isOver()) {
cout << "Game Over!" << endl;
exit(0);
}
for (int i = 0; i < 4; i++) {
Point p = curShape[i];
p.x++;
if (!check(p)) {
updateMap();
checkFull();
newShape();
return;
}
}
for (int i = 0; i < 4; i++) {
curShape[i].x++;
}
}
int main() {
initShapes();
newShape();
while (1) {
refresh();
Sleep(300);
if (_kbhit()) {
int c = _getch();
switch (c) {
case 'a':
moveLeft();
break;
case 'd':
moveRight();
break;
case 'w':
rotate();
break;
case 's':
moveDown();
break;
default:
break;
}
}
else {
moveDown();
}
}
return 0;
}
```
注意:此代码是通过 C++ 开发,需要在 Windows 系统下使用。
阅读全文
相关推荐


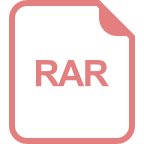
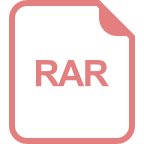
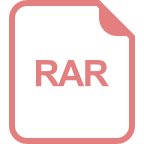
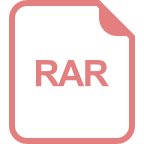
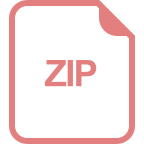
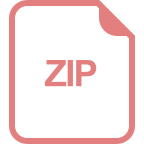
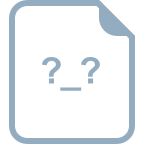
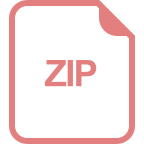
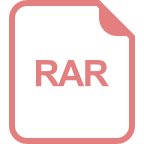
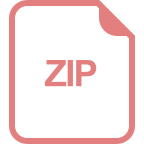
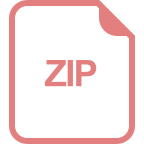
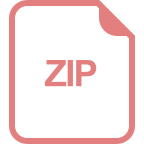
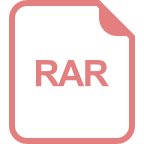
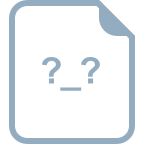