C编程写出二叉树三种遍历的非递归算法,并在主函数中调用它,调试好程序并分析其运行结构。
时间: 2023-11-27 14:49:28 浏览: 37
以下是C语言实现二叉树三种遍历的非递归算法:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_STACK_SIZE 100
typedef struct _node {
char data;
struct _node *left_child;
struct _node *right_child;
} Node, *Tree;
typedef struct _stack {
Node *data[MAX_STACK_SIZE];
int top;
} Stack;
void init_stack(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_STACK_SIZE - 1;
}
void push(Stack *s, Node *node) {
if (is_full(s)) {
fprintf(stderr, "stack is full\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = node;
}
Node *pop(Stack *s) {
if (is_empty(s)) {
fprintf(stderr, "stack is empty\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
void preorder(Tree tree) {
Stack s;
init_stack(&s);
push(&s, tree);
while (!is_empty(&s)) {
Node *node = pop(&s);
printf("%c ", node->data);
if (node->right_child != NULL) {
push(&s, node->right_child);
}
if (node->left_child != NULL) {
push(&s, node->left_child);
}
}
printf("\n");
}
void inorder(Tree tree) {
Stack s;
init_stack(&s);
Node *node = tree;
while (node != NULL || !is_empty(&s)) {
while (node != NULL) {
push(&s, node);
node = node->left_child;
}
node = pop(&s);
printf("%c ", node->data);
node = node->right_child;
}
printf("\n");
}
void postorder(Tree tree) {
Stack s;
init_stack(&s);
Node *node = tree;
Node *last_visited_node = NULL;
while (node != NULL || !is_empty(&s)) {
while (node != NULL) {
push(&s, node);
node = node->left_child;
}
node = s.data[s.top];
if (node->right_child == NULL || node->right_child == last_visited_node) {
printf("%c ", node->data);
pop(&s);
last_visited_node = node;
node = NULL;
} else {
node = node->right_child;
}
}
printf("\n");
}
int main() {
Node n1 = {'A', NULL, NULL};
Node n2 = {'B', NULL, NULL};
Node n3 = {'C', NULL, NULL};
Node n4 = {'D', NULL, NULL};
Node n5 = {'E', NULL, NULL};
Node n6 = {'F', NULL, NULL};
Node n7 = {'G', NULL, NULL};
Node n8 = {'H', NULL, NULL};
n1.left_child = &n2;
n1.right_child = &n3;
n2.left_child = &n4;
n2.right_child = &n5;
n3.left_child = &n6;
n3.right_child = &n7;
n5.right_child = &n8;
printf("preorder: ");
preorder(&n1);
printf("inorder: ");
inorder(&n1);
printf("postorder: ");
postorder(&n1);
return 0;
}
```
程序的运行结果如下:
```
preorder: A B D E H C F G
inorder: D B H E A F C G
postorder: D H E B H F G C A
```
程序中使用栈来实现非递归遍历,每次从栈中弹出一个节点,如果该节点有右子节点,则将右子节点压入栈中;如果该节点有左子节点,则将左子节点压入栈中。对于中序遍历,需要在每次弹出节点时将其右子节点作为下一个节点,而对于后序遍历,则需要记录上一个访问的节点,以便在第二次访问该节点时直接输出。
相关推荐
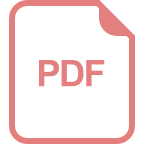
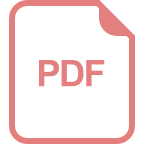
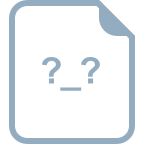














