c语言利用栈完成汗诺塔算法
时间: 2023-07-26 08:21:36 浏览: 56
好的,下面是利用栈完成汉诺塔算法的 C 语言实现示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int n, source, helper, target;
} Problem;
typedef struct {
Problem data[MAX_SIZE];
int top;
} Stack;
void push(Stack *s, Problem p) {
if (s->top == MAX_SIZE - 1) {
printf("Error: stack overflow\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = p;
}
Problem pop(Stack *s) {
if (s->top == -1) {
printf("Error: stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int is_empty(Stack *s) {
return s->top == -1;
}
void hanoi(int n, int source, int helper, int target) {
Stack s;
s.top = -1;
push(&s, (Problem){n, source, helper, target});
while (!is_empty(&s)) {
Problem p = pop(&s);
n = p.n;
source = p.source;
helper = p.helper;
target = p.target;
if (n == 1) {
printf("%d -> %d\n", source, target);
} else {
push(&s, (Problem){n-1, helper, source, target});
push(&s, (Problem){1, source, helper, target});
push(&s, (Problem){n-1, source, target, helper});
}
}
}
int main() {
hanoi(3, 1, 2, 3);
return 0;
}
```
这个算法使用了一个栈来模拟递归调用的过程。每次将需要处理的汉诺塔问题压入栈中,然后根据问题规模的大小,将问题分解为更小的子问题,并将子问题压入栈中。最后,当栈为空时,所有的问题都已经被处理完毕。
相关推荐
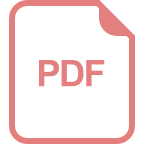














