C语言中写一算法, 将值为x的结点插在链表中第一个值为a的结点之后,如果值为a的结点不存在,则插在表尾。
时间: 2023-09-17 17:05:13 浏览: 76
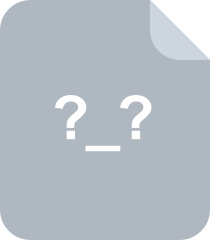
用C语言写的插值算法

### 回答1:
以下是C语言的代码实现:
```
struct Node {
int val;
struct Node* next;
};
void insertNode(struct Node** head, int a, int x) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->val = x;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct Node* curr = *head;
while (curr->next != NULL && curr->val != a) {
curr = curr->next;
}
if (curr->val == a) {
newNode->next = curr->next;
curr->next = newNode;
} else {
curr->next = newNode;
}
}
```
这个算法会遍历链表,找到第一个值为a的结点,然后将值为x的结点插入在它后面。如果值为a的结点不存在,则将值为x的结点插入在表尾。
### 回答2:
要实现这个算法,可以按照以下步骤进行:
1. 定义链表结构和节点结构。链表结构包括一个指向首节点的指针,节点结构包括一个存储值的变量和一个指向下一个节点的指针。
2. 查找值为a的节点,如果找到,则将值为x的节点插入在其后。可以使用一个临时指针遍历链表,找到值为a的节点。
3. 如果找到了值为a的节点,新建一个节点,并将值x赋给新节点的值变量。然后,将原值为a节点的下一个节点指针赋给新节点的下一个节点指针。最后,将新节点的下一个节点指针指向新节点。
4. 如果找不到值为a的节点,则将新节点插入到链表的最后。首先,创建一个临时指针,用于遍历链表直到最后一个节点。然后,将新节点的值赋给新节点的下一个节点指针,并将新节点的下一个节点指针指向空。
下面是具体实现的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构
typedef struct Node {
int value;
struct Node* next;
} Node;
// 定义链表结构
typedef struct List {
Node* head;
} List;
// 将值为x的节点插入链表中
void insertNode(List* list, int a, int x) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = x;
newNode->next = NULL;
Node* temp = list->head;
// 查找值为a的节点
while (temp != NULL && temp->value != a) {
temp = temp->next;
}
if (temp != NULL) {
newNode->next = temp->next;
temp->next = newNode;
} else {
// 找不到值为a的节点,插入到链表末尾
if (list->head == NULL) {
list->head = newNode;
} else {
temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
}
// 测试
int main() {
List list;
list.head = NULL;
// 初始化链表
// 1 -> 2 -> 3 -> 4 -> NULL
for (int i = 1; i <= 4; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = i;
newNode->next = NULL;
if (list.head == NULL) {
list.head = newNode;
} else {
Node* temp = list.head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 插入节点5,值为3的节点之后
// 1 -> 2 -> 3 -> 5 -> 4 -> NULL
insertNode(&list, 3, 5);
// 打印链表
Node* temp = list.head;
while (temp != NULL) {
printf("%d -> ", temp->value);
temp = temp->next;
}
printf("NULL\n");
return 0;
}
```
运行上述代码,输出结果为:1 -> 2 -> 3 -> 5 -> 4 -> NULL。表示成功将值为5的节点插入到值为3的节点之后。
### 回答3:
算法如下:
1. 创建一个指针变量p1指向链表的头节点,并且创建一个指针变量p2指向链表的第一个值为a的节点。
2. 如果p2为NULL,则表示链表中不存在值为a的节点,将新节点插入到链表的尾部。
a. 创建一个新的节点newNode,将新节点的值设置为x,将新节点的下一个节点指向NULL。
b. 如果链表为空,则将新节点设为头节点。
c. 否则,通过遍历链表找到最后一个节点并将其的下一个节点指向新节点。
3. 如果p2不为NULL,则表示链表中存在值为a的节点,将新节点插入到p2节点之后。
a. 创建一个新的节点newNode,将新节点的值设置为x。
b. 将新节点的下一个节点指向p2节点的下一个节点。
c. 将p2节点的下一个节点指向新节点。
4. 返回修改后的链表。
以下是使用C语言编写的算法示例:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int value;
struct Node* next;
};
struct Node* insertNode(struct Node* head, int a, int x) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->value = x;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
struct Node* p1 = head;
struct Node* p2 = head;
while (p2 != NULL && p2->value != a) {
p1 = p2;
p2 = p2->next;
}
if (p2 == NULL) {
p1->next = newNode;
} else {
newNode->next = p2->next;
p2->next = newNode;
}
}
return head;
}
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->value);
current = current->next;
}
}
int main() {
struct Node* head = NULL;
int a, x, n;
printf("请输入链表中的结点数量n:");
scanf("%d", &n);
printf("请输入%d个结点的值:", n);
for (int i = 0; i < n; i++) {
int value;
scanf("%d", &value);
head = insertNode(head, value, value);
}
printf("请输入要插入的结点的值a和x:");
scanf("%d %d", &a, &x);
head = insertNode(head, a, x);
printf("插入后的链表为:");
printList(head);
return 0;
}
```
希望以上回答对您有帮助。
阅读全文
相关推荐















