读取dinosaur.csv恐龙图形的点坐标文件,绘制恐龙图形。(2)变换坐标,x坐标+3, y坐标-2,再绘制新恐龙。,(2)旋转30度角,再绘制新恐龙 (3)旋转60度角,再绘制新恐龙。
时间: 2024-03-17 17:45:00 浏览: 11
好的,这里我假设你已经知道如何读取csv文件并将其转换为点坐标的列表。接下来,我们可以使用Python的turtle库进行绘图操作。代码如下:
```python
import turtle
# 读取csv文件,转换为点坐标列表
points = []
with open('dinosaur.csv', 'r') as f:
for line in f:
x, y = map(float, line.strip().split(','))
points.append((x, y))
# 绘制原恐龙
turtle.penup()
turtle.goto(points[0])
turtle.pendown()
for point in points:
turtle.goto(point)
# 坐标平移变换
new_points = [(x + 3, y - 2) for x, y in points]
# 绘制平移后的恐龙
turtle.penup()
turtle.goto(new_points[0])
turtle.pendown()
for point in new_points:
turtle.goto(point)
# 旋转变换
import math
angle1 = 30 # 旋转角度
angle2 = 60 # 旋转角度
radians1 = math.radians(angle1)
radians2 = math.radians(angle2)
cosine1 = math.cos(radians1)
sine1 = math.sin(radians1)
cosine2 = math.cos(radians2)
sine2 = math.sin(radians2)
new_points1 = [(x * cosine1 - y * sine1, x * sine1 + y * cosine1) for x, y in new_points]
new_points2 = [(x * cosine2 - y * sine2, x * sine2 + y * cosine2) for x, y in new_points]
# 绘制旋转后的恐龙
turtle.penup()
turtle.goto(new_points1[0])
turtle.pendown()
for point in new_points1:
turtle.goto(point)
turtle.penup()
turtle.goto(new_points2[0])
turtle.pendown()
for point in new_points2:
turtle.goto(point)
turtle.done()
```
需要注意的是,turtle库中的goto函数需要传入点的坐标,而不是坐标的元组。因此,我们需要先使用`points[0]`来设置起始点,然后使用`turtle.goto(point)`来依次连接每个点。
在代码中,我们首先绘制了原恐龙,然后进行了坐标平移变换,绘制了平移后的恐龙。接着,我们进行了旋转变换,绘制了旋转后的恐龙。其中,旋转变换使用了三角函数来计算坐标旋转后的值。最后,我们使用`turtle.done()`来保持绘图窗口不关闭,直到手动关闭为止。
相关推荐
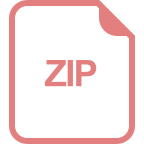
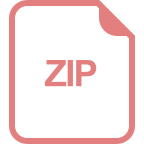















