unity 实现TMP_Text文本末尾光标闪烁,不使用Tmp_inputfield,并且动态改变Tmp_text文本的内容,光标仍然存在
时间: 2024-01-21 16:16:46 浏览: 101
可以通过使用协程来实现末尾光标闪烁的效果。以下是一个简单的示例:
1. 在 Unity 中创建一个空的 GameObject。
2. 将 TMP_Text 组件添加到该 GameObject 上,并设置其文本内容。
3. 创建一个新的 C# 脚本,并将其添加到该 GameObject 上。
4. 在脚本中定义一个 IEnumerator 类型的协程函数,用于控制光标的闪烁。
5. 在 Start 函数中启动该协程,并在 Update 函数中动态修改 TMP_Text 的文本内容即可。
下面是示例代码:
```csharp
using System.Collections;
using TMPro;
using UnityEngine;
public class TextCursorBlink : MonoBehaviour
{
private TMP_Text textComponent;
private Coroutine blinkCoroutine;
private void Start()
{
textComponent = GetComponent<TMP_Text>();
blinkCoroutine = StartCoroutine(BlinkCursor());
}
private void Update()
{
// 动态修改文本内容
textComponent.text = "Hello, World!";
}
private IEnumerator BlinkCursor()
{
while (true)
{
// 在文本末尾添加一个闪烁的光标
textComponent.text += "<color=#00000000>_</color>";
yield return new WaitForSeconds(0.5f);
// 移除光标
textComponent.text = textComponent.text.Remove(textComponent.text.Length - 27);
yield return new WaitForSeconds(0.5f);
}
}
}
```
在上述代码中,我们使用了 TMP_Text 的 rich text 标记 `<color>` 来添加一个黑色的光标,并使用协程来控制光标的闪烁。在动态修改文本内容时,光标仍然存在。
阅读全文
相关推荐
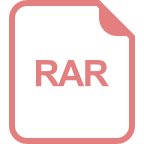
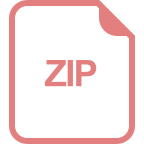




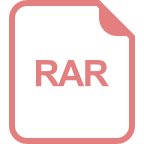
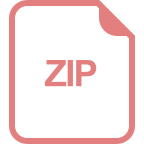
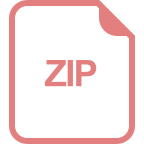
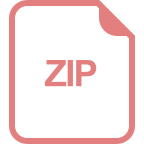
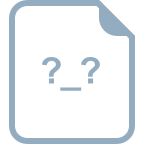
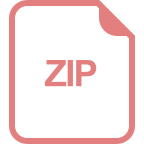
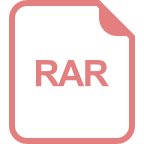
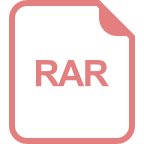
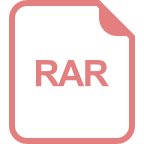
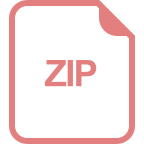
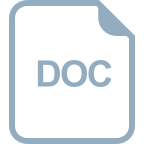