Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。所有顶点读完之后再读取面片信息,根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中。为了方便Polyhedron对Facet和Point的数据成员进行操作,可以让Facet和Point类将Polyhedron设置为friend类。在Polyhedron的析构函数中需要完成对所有Facet和Point的析构。先遍历facets中的所有面片进行delete,再遍历vertices中的所有Point进行delete。保证所有new出来的对象都被delete了。写程序
时间: 2024-02-11 17:05:28 浏览: 61
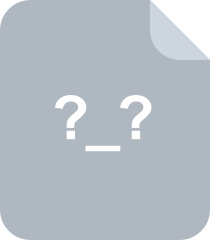
Polyhedron函数测试代码
以下是Polyhedron类的实现,包括构造函数和析构函数:
```c++
#include <fstream>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
template<typename T>
class Point;
template<typename T>
class Facet;
template<typename T>
class Polyhedron {
public:
Polyhedron(const char* path) {
ifstream fin(path);
if (!fin) {
cerr << "Failed to open file: " << path << endl;
return;
}
string line;
getline(fin, line);
if (line != "OFF") {
cerr << "Invalid file format: " << path << endl;
return;
}
getline(fin, line);
istringstream iss(line);
int vertexCount, facetCount;
iss >> vertexCount >> facetCount;
for (int i = 0; i < vertexCount; i++) {
T x, y, z;
fin >> x >> y >> z;
Point<T>* p = new Point<T>(x, y, z);
vertices.push_back(p);
}
for (int i = 0; i < facetCount; i++) {
int vertexIndexCount;
fin >> vertexIndexCount;
Facet<T>* f = new Facet<T>();
for (int j = 0; j < vertexIndexCount; j++) {
int vertexIndex;
fin >> vertexIndex;
f->vertices.push_back(vertices[vertexIndex]);
}
facets.push_back(f);
}
fin.close();
}
~Polyhedron() {
for (auto f : facets) {
delete f;
}
for (auto p : vertices) {
delete p;
}
}
friend class Facet<T>;
friend class Point<T>;
private:
vector<Point<T>*> vertices;
vector<Facet<T>*> facets;
};
template<typename T>
class Point {
public:
Point(T x, T y, T z) : x(x), y(y), z(z) {}
friend class Polyhedron<T>;
private:
T x, y, z;
};
template<typename T>
class Facet {
public:
friend class Polyhedron<T>;
private:
vector<Point<T>*> vertices;
};
```
在上述代码中,Polyhedron类的构造函数实现了对OFF文件的读取,通过逐个读取文件中的数据并new出对应的Point和Facet对象,最终构造出多面体。在构造函数中,还需要检查文件是否成功打开,以及OFF文件格式是否正确。Polyhedron类的析构函数则完成了对所有Point和Facet对象的释放。为了方便Polyhedron对Facet和Point的数据成员进行操作,我们将Facet和Point类设置为Polyhedron的友元类。
注意,由于Polyhedron类中保存了许多指针,我们需要确保在程序结束时显式地释放它们,否则会出现内存泄漏的问题。
阅读全文
相关推荐
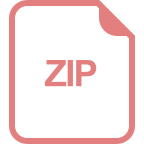
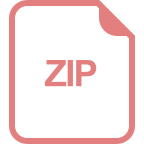





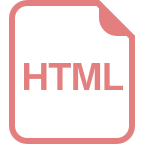