用C语言写一个用户输入顶点信息有向图并遍历的程序,要求能实现两种遍历方式
时间: 2024-03-26 12:40:31 浏览: 17
好的,我来为您编写一个用C语言实现有向图遍历的程序。程序会让用户输入图的顶点信息,并根据用户输入创建有向图。然后,程序会实现深度优先遍历和广度优先遍历两种遍历方式。
下面是程序的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_VERTICES 100
typedef struct Vertex {
char name;
int id;
int visited;
struct Edge* edges;
} Vertex;
typedef struct Edge {
struct Vertex* dest;
struct Edge* next;
} Edge;
typedef struct Graph {
int numVertices;
struct Vertex* vertices;
} Graph;
Edge* newEdge(Vertex* dest, Edge* next) {
Edge* edge = (Edge*)malloc(sizeof(Edge));
edge->dest = dest;
edge->next = next;
return edge;
}
Vertex* newVertex(char name, int id) {
Vertex* vertex = (Vertex*)malloc(sizeof(Vertex));
vertex->name = name;
vertex->id = id;
vertex->visited = false;
vertex->edges = NULL;
return vertex;
}
void addEdge(Graph* graph, int src, int dest) {
Vertex* srcVertex = &(graph->vertices[src]);
Edge* edge = newEdge(&(graph->vertices[dest]), srcVertex->edges);
srcVertex->edges = edge;
}
Graph* newGraph(int numVertices) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->numVertices = numVertices;
graph->vertices = (Vertex*)malloc(sizeof(Vertex) * numVertices);
for (int i = 0; i < numVertices; i++) {
graph->vertices[i] = *newVertex('\0', i);
}
return graph;
}
void dfs(Vertex* vertex) {
vertex->visited = true;
printf("%c ", vertex->name);
Edge* edge = vertex->edges;
while (edge != NULL) {
if (!edge->dest->visited) {
dfs(edge->dest);
}
edge = edge->next;
}
}
void bfs(Vertex* vertex) {
int front = 0;
int rear = 0;
Vertex* queue[vertex->visited + 1];
queue[rear++] = vertex;
vertex->visited = true;
printf("%c ", vertex->name);
while (front != rear) {
Vertex* v = queue[front++];
Edge* edge = v->edges;
while (edge != NULL) {
if (!edge->dest->visited) {
edge->dest->visited = true;
printf("%c ", edge->dest->name);
queue[rear++] = edge->dest;
}
edge = edge->next;
}
}
}
int main() {
int numVertices;
printf("请输入图中顶点的数量:");
scanf("%d", &numVertices);
Graph* graph = newGraph(numVertices);
char vertexName;
for (int i = 0; i < numVertices; i++) {
printf("请输入第%d个顶点的名称:", i+1);
scanf(" %c", &vertexName);
graph->vertices[i].name = vertexName;
}
int numEdges;
printf("请输入图中边的数量:");
scanf("%d", &numEdges);
int src, dest;
for (int i = 0; i < numEdges; i++) {
printf("请输入第%d条边的起点和终点的编号(用空格隔开):", i+1);
scanf("%d %d", &src, &dest);
addEdge(graph, src, dest);
}
printf("深度优先遍历结果:");
for (int i = 0; i < graph->numVertices; i++) {
if (!graph->vertices[i].visited) {
dfs(&(graph->vertices[i]));
}
}
printf("\n");
for (int i = 0; i < graph->numVertices; i++) {
graph->vertices[i].visited = false;
}
printf("广度优先遍历结果:");
for (int i = 0; i < graph->numVertices; i++) {
if (!graph->vertices[i].visited) {
bfs(&(graph->vertices[i]));
}
}
printf("\n");
return 0;
}
```
该程序会先要求用户输入图的顶点数量,然后根据顶点数量创建图。接着,程序会要求用户输入每个顶点的名称,并将每个顶点的名称存储在对应的Vertex结构体中。然后,程序会要求用户输入图的边数量,并根据用户输入创建图的边。最后,程序将实现深度优先遍历和广度优先遍历,并输出遍历结果。
注意:在读取顶点名称时,需要在scanf函数前加一个空格,否则会出现预期之外的问题。
相关推荐
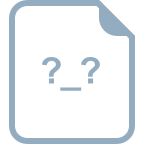
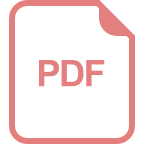
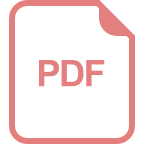
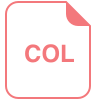













