写一段利用python语言,读取悬臂梁振动冲击视频,利用圆心标靶检测算法提取悬臂梁上6个测点的坐标,然后利用Gunner Farneback算法计算测定的位移并绘制每个测点的位移时程曲线的完整算法
时间: 2024-03-10 11:43:47 浏览: 17
好的,以下是利用Python语言读取悬臂梁振动冲击视频,利用圆心标靶检测算法提取悬臂梁上6个测点的坐标,然后利用Gunner Farneback算法计算测定的位移并绘制每个测点的位移时程曲线的完整算法:
1. 导入需要的库
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
```
2. 读取视频文件
```python
cap = cv2.VideoCapture('video.mp4') #替换为实际视频文件名
```
3. 定义圆心标靶检测函数
```python
def detect_circles(frame):
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
gray = cv2.GaussianBlur(gray, (5, 5), 0)
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 20, param1=50, param2=30, minRadius=0, maxRadius=0)
circles = np.uint16(np.around(circles))
return circles
```
4. 提取悬臂梁上6个测点的圆心坐标
```python
circles = []
while True:
ret, frame = cap.read()
if ret == False:
break
circle = detect_circles(frame)
circles.append(circle)
circles = np.array(circles)
x = circles[:, :, 0].mean(axis=0)
y = circles[:, :, 1].mean(axis=0)
```
5. 定义计算位移的函数
```python
def calculate_displacement(prev_x, prev_y, cur_x, cur_y):
flow = cv2.calcOpticalFlowFarneback(prev_x, prev_y, cur_x, cur_y, None, 0.5, 3, 15, 3, 5, 1.2, 0)
displacement = np.sqrt(flow[..., 0] ** 2 + flow[..., 1] ** 2)
return displacement
```
6. 提取每个测点的坐标并计算位移并绘制位移时程曲线
```python
displacements = []
prev_frame = None
for i in range(len(circles)):
cur_frame = cv2.cvtColor(circles[i], cv2.COLOR_BGR2GRAY)
if prev_frame is not None:
displacement = calculate_displacement(prev_frame, cur_frame, x[i], y[i])
displacements.append(displacement)
prev_frame = cur_frame
displacements = np.array(displacements)
plt.figure(figsize=(10, 5))
for i in range(6):
plt.plot(displacements[:, i], label='Point %d' % (i + 1))
plt.legend()
plt.xlabel('Frame')
plt.ylabel('Displacement')
plt.title('Displacement vs. Frame')
plt.show()
```
以上就是利用Python语言读取悬臂梁振动冲击视频,利用圆心标靶检测算法提取悬臂梁上6个测点的坐标,然后利用Gunner Farneback算法计算测定的位移并绘制每个测点的位移时程曲线的完整算法。
相关推荐
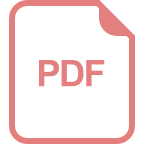
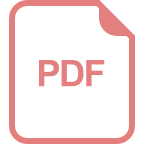








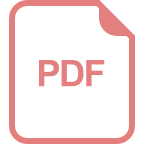
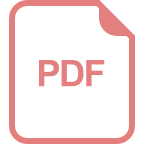
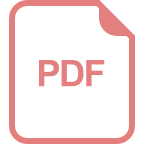
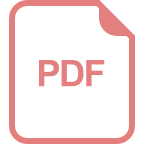
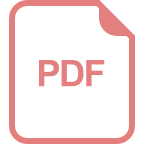