写一段利用python语言,读取悬臂梁振动冲击视频,利用圆心标靶检测算法提取悬臂梁上6个测点,并使用Lucas-Kanade光流法对测点进行跟踪并计算位移,将每个测点的位移数据存储下来,并绘制位移时程曲线的完整代码
时间: 2023-12-23 09:02:51 浏览: 37
好的,以下是一个基本的代码框架,可以实现您所需功能:
```
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 读取视频
cap = cv2.VideoCapture('your_video_path')
# 定义圆形检测函数
def detect_circles(frame):
# 在此处编写HoughCircles检测圆形的代码
return circles
# 定义Lucas-Kanade光流法函数
def lk_flow(prev, curr, points):
# 在此处编写Lucas-Kanade光流法的代码
return points, new_points, status, errors
# 定义存储位移数据的字典
displacement = {'Point1': [], 'Point2': [], 'Point3': [], 'Point4': [], 'Point5': [], 'Point6': []}
# 循环读取视频帧
while True:
ret, frame = cap.read()
if not ret:
break
# 灰度化
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 圆形检测
circles = detect_circles(gray)
# 提取6个测点
points = [] # 存储测点的位置
for circle in circles:
x, y, r = circle[0], circle[1], circle[2]
# 在此处编写确定测点位置的代码
points.append((x, y))
# Lucas-Kanade光流法跟踪测点并计算位移
if len(points) > 0:
if 'prev_gray' not in locals():
prev_gray = gray.copy()
prev_points = np.array(points, dtype=np.float32)
else:
curr_gray = gray.copy()
curr_points, status, errors = lk_flow(prev_gray, curr_gray, prev_points)
for i, p in enumerate(curr_points):
if status[i] == 1:
# 计算位移并存储
dx = curr_points[i][0] - prev_points[i][0]
dy = curr_points[i][1] - prev_points[i][1]
displacement['Point' + str(i + 1)].append((dx, dy))
# 更新上一帧的图像和测点
prev_gray = curr_gray.copy()
prev_points = curr_points.copy()
# 绘制位移时程曲线
plt.figure(figsize=(10, 8))
for key, value in displacement.items():
x, y = zip(*value)
plt.plot(x, label=key)
plt.legend()
plt.xlabel('Frame')
plt.ylabel('Displacement')
plt.show()
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
需要注意的是,该代码仅为基本框架,具体实现需要根据您的数据和环境进行调整和优化。另外,圆形检测和Lucas-Kanade光流法的具体实现可以参考OpenCV官方文档或其他相关资料。
相关推荐
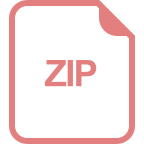
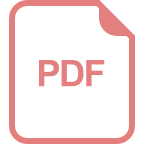








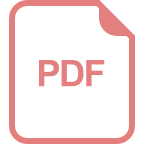
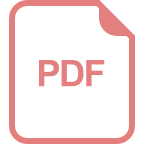
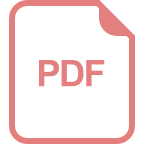
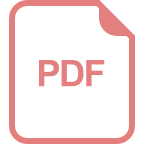
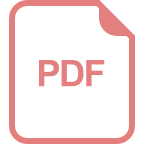
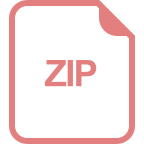