6.创建一个包含多个对象的数组,每个对象包含书名、作者和阅读状态信息。实现 显示所有书籍和设置书籍阅读状态的功能。
时间: 2024-09-27 08:04:48 浏览: 10
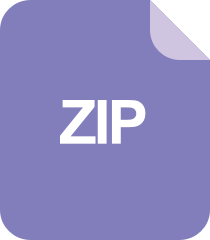
remindme:一个简单的基于提醒的应用程序,可以跟踪书籍和电影
为了创建一个包含多个对象的数组,每个对象表示一本书,你可以这样做:
```javascript
// 定义一个Book类或对象构造函数
class Book {
constructor(title, author, readingStatus = false) {
this.title = title;
this.author = author;
this.readingStatus = readingStatus; // 默认为未读
}
// 可选的方法:显示书籍详细信息
displayDetails() {
console.log(`Title: ${this.title}, Author: ${this.author}, Reading Status: ${this.readingStatus}`);
}
}
// 创建一个包含多本书的数组
let library = [
new Book("The Catcher in the Rye", "J.D. Salinger"),
new Book("To Kill a Mockingbird", "Harper Lee"),
new Book("Pride and Prejudice", "Jane Austen")
];
// 显示所有书籍
library.forEach(book => book.displayDetails());
// 设置书籍阅读状态
function setReadingStatus(booksArray, index, status) {
if (index >= 0 && index < booksArray.length) {
booksArray[index].readingStatus = status;
booksArray[index].displayDetails();
} else {
console.log("Invalid index");
}
}
// 示例:设置第一本书为已读
setReadingStatus(library, 0, true);
```
在这个例子中,我们首先定义了一个`Book`类,包含了书名、作者和阅读状态属性。然后创建了一个包含三个实例的数组`library`。我们提供了一个`displayDetails()`方法用于显示书籍信息,以及一个`setReadingStatus()`函数用于设置书籍的阅读状态。
要查看所有书籍,我们可以遍历数组并调用每个对象的`displayDetails()`方法。设置阅读状态时,我们传递数组、索引和新状态给`setReadingStatus()`函数。
阅读全文
相关推荐
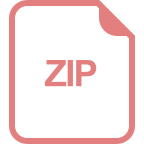
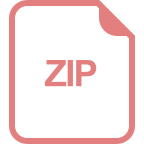




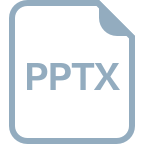
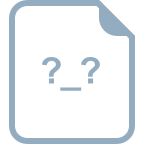
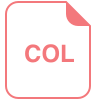
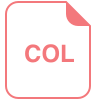







