八皇后问题java游戏代码
时间: 2023-12-20 12:28:32 浏览: 34
以下是八皇后问题的Java代码实现,使用回溯算法:
```
public class EightQueens {
private static final int BOARD_SIZE = 8;
private static int[] queens = new int[BOARD_SIZE];
public static void main(String[] args) {
placeQueens(0);
}
private static void placeQueens(int row) {
if (row == BOARD_SIZE) {
printQueens();
return;
}
for (int col = 0; col < BOARD_SIZE; col++) {
if (isValidPosition(row, col)) {
queens[row] = col;
placeQueens(row + 1);
}
}
}
private static boolean isValidPosition(int row, int col) {
for (int i = 0; i < row; i++) {
int diff = Math.abs(col - queens[i]);
if (diff == 0 || diff == row - i) {
return false;
}
}
return true;
}
private static void printQueens() {
for (int row = 0; row < BOARD_SIZE; row++) {
for (int col = 0; col < BOARD_SIZE; col++) {
if (queens[row] == col) {
System.out.print("Q ");
} else {
System.out.print(". ");
}
}
System.out.println();
}
System.out.println(); }
}
```
这个程序使用一个一维数组来表示棋盘上每一行皇后的位置,数组的下标表示行数,数组元素的值表示列数。在placeQueens方法中,程序在当前列选择一个可以放置皇后的行,如果找到了一组合法解,就打印出来,否则回溯到上一步继续寻找。isValidPosition方法用于判断当前位置是否可以放置皇后,printQueens方法用于打印出一组合法解。
相关推荐
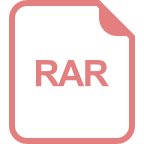














