【从零开始学习回溯】:Java代码实践与算法思维的双重培养
发布时间: 2024-08-29 21:24:23 阅读量: 20 订阅数: 36 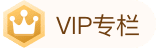
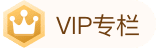
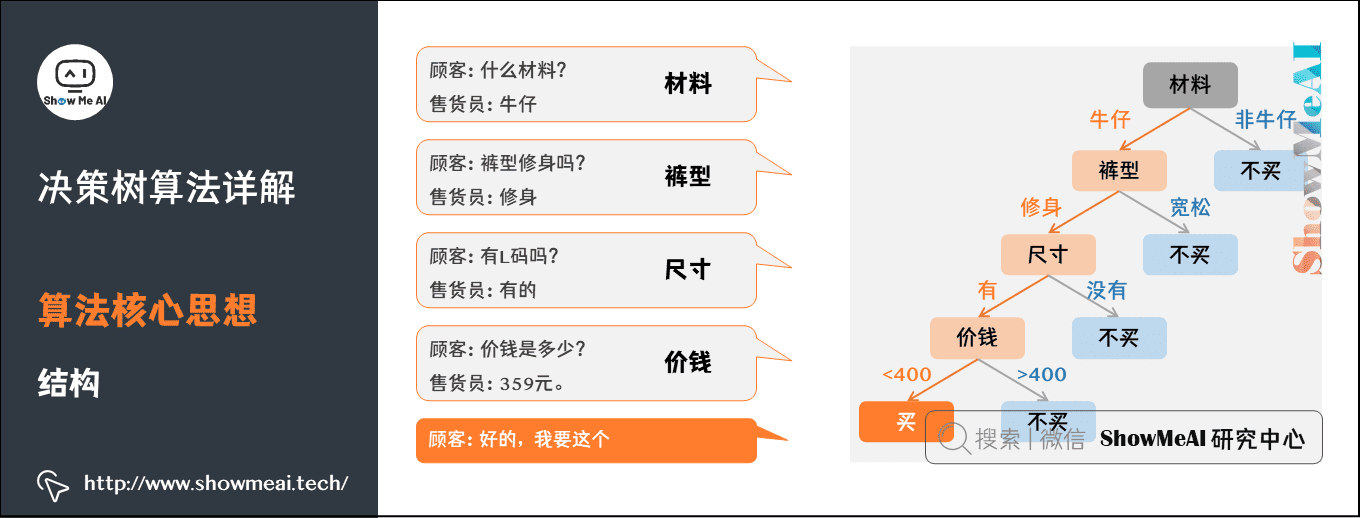
# 1. 回溯算法的概念与应用
在计算机科学中,回溯算法是一类通过试错来寻找问题答案的算法,它会遍历所有可能性,当发现当前路径不可能达到有效解时,会回退到上一步并尝试其他可能的路径。这种方法在解决组合问题、排列问题以及某些约束满足问题时尤为有效。例如,在解决N皇后问题时,回溯算法可以用来放置N个皇后,确保它们不相互攻击,即任意两个皇后都不在同一行、同一列或同一对角线上。本章将首先介绍回溯算法的基本概念,并探讨其在现实世界中的应用场景,以帮助读者理解回溯算法的重要性和实用性。
# 2. ```
# 第二章:Java基础与回溯算法的关系
Java是编写回溯算法的理想选择,因其具备了面向对象的特性,丰富的API以及强大的集合框架。在深入探讨回溯算法之前,让我们先来回顾Java的基础知识,并了解其如何与回溯算法相结合。
## 2.1 Java基本语法回顾
### 2.1.1 数据类型和变量
Java语言是一种强类型语言,在Java中,每个变量和表达式都有一个类型,必须声明后使用。基本数据类型包括了数值型、字符型、布尔型等。整数类型有byte、short、int、long;浮点类型有float、double;字符类型为char;布尔类型为boolean。
变量是存储数据的基本单元,在Java中变量的定义必须指定类型。例如:
```java
int number = 10; // 定义一个整型变量number,并赋值为10
boolean flag = true; // 定义一个布尔型变量flag,并赋值为true
```
### 2.1.2 控制流程语句
Java使用控制流程语句来控制程序的执行流程。这些语句包括了选择语句(if-else、switch)和循环语句(for、while、do-while)。选择语句根据条件表达式的真假来选择性地执行不同的代码块。循环语句则可以重复执行某些代码直到满足特定条件。
示例代码:
```java
if (number > 0) {
flag = true; // 如果number大于0,则设置flag为true
} else {
flag = false; // 否则设置flag为false
}
for (int i = 0; i < number; i++) {
System.out.println("This is loop iteration: " + i); // 循环打印
}
```
## 2.2 Java面向对象编程
### 2.2.1 类与对象
面向对象编程(OOP)是Java的核心概念之一。类是创建对象的模板,对象是类的实例。在Java中,使用关键字`class`定义类。创建类的对象使用`new`关键字。
示例代码:
```java
public class Car {
String color; // 类的属性
int numberOfWheels; // 类的属性
public Car(String color, int numberOfWheels) { // 构造函数
this.color = color;
this.numberOfWheels = numberOfWheels;
}
void startEngine() { // 类的方法
System.out.println("Engine started.");
}
}
// 创建Car类的对象
Car myCar = new Car("red", 4);
myCar.startEngine();
```
### 2.2.2 继承与多态
继承允许创建一个类,该类继承了另一个类的属性和方法,提高了代码的重用性。Java中使用`extends`关键字实现继承。多态是指允许不同类的对象对同一消息做出响应的能力。
示例代码:
```java
class Vehicle {
void start() {
System.out.println("Vehicle is starting.");
}
}
class Motorcycle extends Vehicle {
@Override
void start() {
System.out.println("Motorcycle is starting with a roar.");
}
}
class Truck extends Vehicle {
@Override
void start() {
System.out.println("Truck is starting with heavy noise.");
}
}
Vehicle vehicle = new Motorcycle();
vehicle.start(); // 输出: Motorcycle is starting with a roar.
```
## 2.3 Java集合框架与算法实现
### 2.3.1 集合框架概述
Java集合框架为表示和操作集合提供了一套设计良好的支持接口和实现类。它由一组接口和类组成,可以用来存储对象的集合,并且定义了操作集合的方法。常用的数据结构如List、Set和Map。
### 2.3.2 集合在回溯算法中的应用
在回溯算法中,集合常用于存储中间状态,例如,可以使用List来存储一个路径或序列。Java集合框架中提供的数据结构为算法提供了强大的辅助支持,使得算法设计更加简洁高效。
示例代码:
```java
import java.util.List;
import java.util.ArrayList;
public class BacktrackingExample {
public List<List<Integer>> subsets(int[] nums) {
List<List<Integer>> results = new ArrayList<>();
backtrack(nums, 0, new ArrayList<>(), results);
return results;
}
private void backtrack(int[] nums, int start, List<Integer> current, List<List<Integer>> results) {
results.add(new ArrayList<>(current)); // 将当前状态保存
for (int i = start; i < nums.length; i++) {
current.add(nums[i]); // 添加一个元素
backtrack(nums, i + 1, current, results); // 继续回溯
current.remove(current.size() - 1); // 回溯中恢复状态
}
}
}
```
以上内容回顾了Java的基本语法,包括数据类型、变量、控制流程语句,面向对象编程的相关概念,如类、对象、继承和多态,以及Java集合框架的基本使用,为后续章节中回溯算法的实现打下了坚实的基础。
```
# 3. 回溯算法的Java实现
## 3.1 回溯算法的理论基础
### 3.1.1 算法描述与伪代码
回溯算法是一种通过探索所有潜在可能性来找到所有解决方案的算法。当它遇到一个解决方案时,它回退并尝试另一个可能的解决方案。回溯算法常用于解决约束满足问题,比如数独、组合、排列问题等。
伪代码描述回溯算法的基本结构如下:
```
function backtrack(路径, 选择列表):
if 满足结束条件:
存储路径
return
for 选择 in 选择列表:
做出选择
如果合法:
backtrack(路径, 新的选择列表)
撤销选择
```
这个结构体现了回溯算法的递归本质,它尝试每一种可能的选择,并在达到解或无解时回溯。
### 3.1.2 算法的时间复杂度分析
回溯算法的时间复杂度取决于问题的规模和解决方案的数量。在最坏的情况下,回溯算法可能需要遍历整个搜索空间。对于某些问题,这个搜索空间可以非常巨大,导致时间复杂度是指数级的。对于组合问题,时间复杂度通常为 O(n!),其中 n 是问题的规模。对于排列问题,时间复杂度可以达到 O(n*n!)。
## 3.2 回溯算法的Java代码模板
### 3.2.1 递归函数的构建
回溯算法的实现通常涉及递归函数。递归函数通过不断将问题分解为更小的子问题,并在找到解决方案或无解时回溯。
```java
public void backtrack(int[] nums, List<Integer> path, List<List<Integer>> results) {
// 满足结束条件,存储路径
if (满足结束条件) {
results.add(new ArrayList<>(path));
return;
}
// 尝试每一种选择
for (int i = 0; i < nums.length; i++) {
// 做出选择
path.add(nums[i]);
// 进行下一步的递归操作
backtrack(nums, path, results);
// 撤销选择
path.remove(path.size() - 1);
}
}
```
### 3.2.2 状态保存与恢复机制
在回溯过程中,状态保存与恢复机制对于提高效率非常关键。这通常涉及到对变量的保存与恢复,以防止重复计算和错误回溯。
```java
public void backtrack(List<Integer> state) {
// 保存当前状态
int previousState = ...;
// 尝试新的选择
for (int i = 0; i
```
0
0
相关推荐
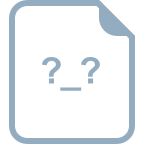
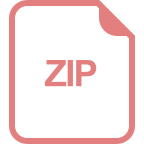
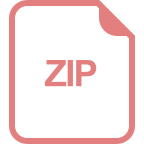





