【Java回溯算法详解】:掌握迷宫、八皇后等经典问题的解决方案与优化
发布时间: 2024-08-29 21:29:04 阅读量: 57 订阅数: 36 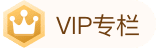
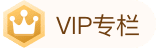
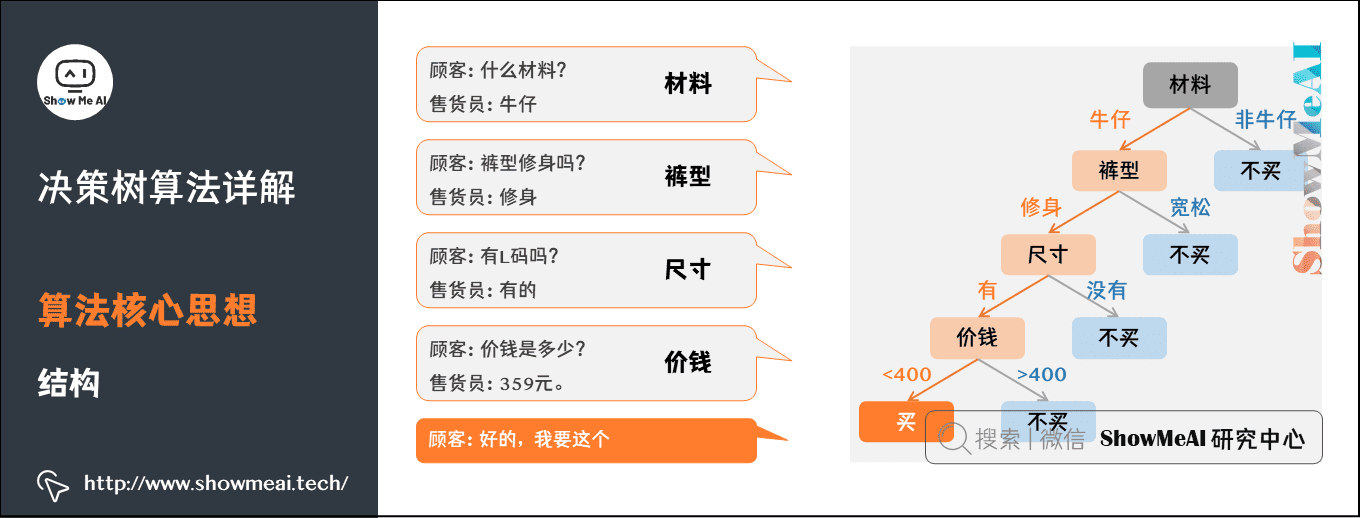
# 1. Java回溯算法的原理与应用
在探索编程的海洋中,算法始终是我们的罗盘。本章将带您深入理解Java回溯算法,这是一种通过递归方式解决组合问题的经典算法。我们将从它的基本原理入手,探讨其在不同领域的应用,从而为后续章节的内容打下坚实基础。
## 1.1 回溯算法的定义
回溯算法是一种通过探索所有可能的候选解来找出所有解的算法。当它发现一个候选解不可能成为解时,会放弃当前的线索,回溯到上一步,然后尝试新的候选解。
## 1.2 回溯算法的基本思想
核心思想可以概括为“尝试-回溯”。它按照一定的顺序,尝试每个可能的候选解,一旦发现当前候选解不可能满足条件时,就放弃此解,回溯到上一个解继续尝试。
## 1.3 回溯算法的应用场景
在现实世界中,回溯算法广泛应用于各种需要全排列或组合的场景,如旅行推销员问题、八皇后问题、图的着色问题、数独等。在实际应用中,根据问题的不同,我们可能需要对基本的回溯算法进行适当的调整和优化,以提高效率。
理解了回溯算法的基本原理后,接下来章节将介绍如何在Java中掌握和应用回溯算法。
# 2. 掌握Java回溯算法的基本框架
### 2.1 回溯算法的基本概念
#### 2.1.1 算法的定义和核心思想
回溯算法是一种通过递归来遍历或者搜索所有可能情况的算法,它是一种既包含系统性又包含试探性的搜索过程。在算法执行过程中,当它通过尝试发现现有的分步决策不能得到有效的解答时,就取消上一步甚至上几步的计算,再通过其他的决策再次尝试寻找问题的答案。
核心思想是:在每一步选择中都尝试可能性,一旦发现当前选择不是一个正确的选择(即当前解不是可行解),就回退到上一步进行其他尝试。这种策略在问题规模较大时非常有效,因为它能够大量地剪枝,避免无效的搜索。
#### 2.1.2 算法的结构框架
回溯算法通常具有以下结构框架:
- 定义一个递归函数,该函数包含决策变量列表、当前路径、决策列表等状态。
- 在递归函数中,按照某种顺序对决策变量进行尝试。
- 每次尝试之前检查是否满足约束条件,若不满足则跳过。
- 若当前尝试产生了一个可行解,将其记录下来或进行进一步处理。
- 当所有变量都尝试完毕后,若有解则返回成功,无解则回溯到上一级决策继续尝试其他可能。
- 回溯至最上层,结束搜索。
### 2.2 Java回溯算法的关键技术
#### 2.2.1 递归技术的使用
递归是回溯算法中不可或缺的技术。在Java中,递归函数需要一个终止条件和继续递归的条件。下面是递归的基本代码模式:
```java
public void recur(int level, int param) {
// 递归终止条件
if (level > MAX_LEVEL) {
return;
}
// 处理当前层逻辑
// 递归的下一步
recur(level + 1, newParam);
// 回溯时的清理工作
}
```
在这个函数中,`level`是递归深度,`param`是当前层处理的数据。递归终止条件是达到最大深度`MAX_LEVEL`。每一层的处理逻辑在函数体中体现,而在回溯之前需要进行清理,以保证状态的正确性。
#### 2.2.2 状态维护和变量回溯
在回溯算法中,状态维护指的是记录每一层决策所做选择的状态。变量回溯是通过撤销上一层的选择,以保证算法能够遍历所有可能的情况。以下是变量回溯的简单实现:
```java
int[] result = new int[MAX_LEVEL];
int level = 0;
public void backtrack(int[] result, int level) {
if (level == MAX_LEVEL) {
// 达到解
printResult(result);
return;
}
for (int i = 0; i < MAX_OPTION; i++) {
result[level] = i; // 尝试第i个选项
// 检查是否满足约束
if (isValid(result, level)) {
backtrack(result, level + 1); // 进入下一层
}
// 回溯前的清理工作(状态重置)
result[level] = 0;
}
}
```
#### 2.2.3 剪枝策略和性能优化
剪枝是回溯算法中非常重要的优化策略,它指的是在搜索过程中尽早地放弃当前的搜索分支。这可以通过增加额外的约束条件来实现,以减少不必要的计算。
```java
// 剪枝策略示例
public void backtrack(int[] result, int level) {
if (level == MAX_LEVEL) {
printResult(result);
return;
}
for (int i = 0; i < MAX_OPTION; i++) {
result[level] = i;
// 检查是否满足剪枝条件
if (isPruned(result, level)) {
continue;
}
if (isValid(result, level)) {
backtrack(result, level + 1);
}
result[level] = 0;
}
}
```
在这段代码中,`isPruned`是一个剪枝函数,它通过某种规则判断当前分支是否值得继续探索。如果当前选择会导致无解,则直接跳过当前分支,不进行递归。
### 2.3 Java回溯算法的案例分析
#### 2.3.1 N皇后问题的解法
N皇后问题是一个经典的回溯问题。问题的目标是在N×N的棋盘上放置N个皇后,使得它们不能相互攻击。攻击的定义是任意两个皇后都不在同一行、同一列、同一斜线上。
实现步骤如下:
1. 初始化棋盘,可以使用一维数组`int[] board = new int[n];`来表示。
2. 从第1行开始,依次尝试在每一行的每一列放置皇后。
3. 检查当前位置是否安全,可以通过判断同一列和同一斜线上是否已有皇后。
4. 如果当前放置皇后的位置安全,则记录下来,并继续尝试下一行的放置。
5. 如果不安全,则回溯到上一行,移动皇后到下一个位置。
6. 重复以上步骤,直到所有皇后都放置完毕,输出解决方案。
#### 2.3.2 迷宫寻路问题的解法
迷宫寻路问题要求找到从迷宫的一个入口到达出口的路径。迷宫通常用二维数组表示,其中0表示可以走的路,1表示障碍。
回溯算法的编码实现步骤:
1. 初始化路径,使用一个二维数组`boolean[][] visited`来标记是否走过。
2. 从迷宫的入口开始,尝试每一条可能的路径。
3. 每次移动前检查当前位置是否可以走,如果可以走,则标记为已访问。
4. 如果当前位置不能走,则回溯到上一个位置。
5. 当成功到达出口时,输出路径或者路径长度。
6. 如果当前位置无法继续移动,并且回溯到入口后仍没有找到出路,则表示无解。
本章节我们详细介绍了回溯算法在Java中实现的基本框架和关键技术,以及案例分析。下章节将深入探讨Java回溯算法在经典问题中的解决方案,包括八皇后、迷宫问题、图的着色等,同时还会涉及如何优化回溯算法,提高效率。
# 3. 经典问题的Java回溯实现与优化
在IT行业中,算法是解决问题的核心工具,尤其是对于需要穷举大量可能性的经典问题,如八皇后问题、迷宫问题、图的着色问题等。Java回溯算法以其对复杂问题的高效处理能力,成为了这一领域的宠儿。本章将深入探讨这些经典问题的Java回溯实现,以及如何通过优化提升算法的性能。
## 3.1 八皇后问题的解决方案
八皇后问题要求在8×8的棋盘上放置八个皇后,使它们互不攻击,即任意两个皇后都不在同一行、同一列或同一斜线上。这是一个典型的NP完全问题,回溯算法是其经典解法。
### 3.1.1 问题描述和挑战
问题的关键在于如何设计一个有效的回溯算法,它需要考虑如何表示棋盘状态,如何检测皇后之间的冲突,以及如何高效地遍历所有可能的棋盘配置。
### 3.1.2 回溯算法的实现步骤
实现八皇后问题的回溯算法主要步骤如下:
1. 从第一行开始,尝试在每一列放置皇后。
2. 检查放置皇后的位置是否安全(不与其他皇后冲突)。
3. 如果当前位置安全,则递归地将问题规模缩小,即在下一行放置另一个皇后。
4. 如果到达最后一行且放置了皇后,则记录当前解。
5. 回溯至前一行,移动皇后到下一个安全的位置,重复步骤2-4。
6. 当所有行都尝试过,所有皇后都放置完毕,结束搜索。
```java
public class EightQueens {
private final int size = 8; // 棋盘大小
private int[] board = new int[size]; // 棋盘,索引表示行,值表示列
private List<int[]> solutions = new ArrayList<>(); // 存储所有解的列表
public void solve() {
placeQueen(0);
printSolutions();
}
private void placeQueen(int row) {
if (row == size) {
solutions.add(Arrays.copyOf(board, size));
return;
}
for (int col = 0; col < size; col++) {
if (isSafe(row, col)) {
board[row] = col;
placeQueen(row + 1);
}
}
}
private boolean isSafe(int row, int col) {
for (int i = 0; i < row; i++) {
if (board[i] == col || Math.abs(row - i) == Math.abs(col - board[i])) {
return false;
}
}
return true;
}
private void printSolutions() {
for (int[] solution : solutions) {
for (int col : solution) {
System.out.print("Q ");
}
System.out.println();
System.out.println();
}
}
}
```
### 3.1.3 优化技巧和效率提升
八皇后问题可以通过一些优化技巧显著提高效率:
- **剪枝策略**:在回溯算法的遍历过程中,当某一行的某一列不能放置皇后时,无需继续考虑该行之后的列,可以立即剪枝。
- **位运算**:使用位运算来表示棋盘状态,可以减少不必要的数组操作,提高访问速度。
通过这些优化,算法在遍历过程中可以跳过大量无效状态,从而提高整体的搜索效率。
## 3.2 迷宫问题的解决方案
迷宫问题要求在一个给定的二维数组中,找出从起点到终点的路径
0
0
相关推荐
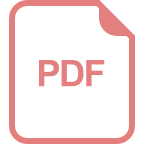
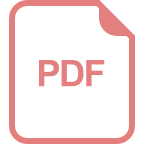





