用C语言设计一个对n个并发进程进行调度的程序,每个进程由一个进程控制块(PCB)结构表示,该进程控制块应包括下述信息:进程标识ID、进程优先数PRIORITY(并规定优先数与优先权成正比)、时间片数CHIP、进程已经占用CPU的时间CPUTIME,进程还需要运行的时间ALLTIME(当进程运行完毕时,其值为0)、进程的状态STATE(为简化起见。设每个进程处于运行E(excecuting)、就绪R(ready)和完成F(finish)三种状态之一,并假设起始状态都是就绪状态R。),以及进程队列指针NEXT(用来将PCB排成队列)等,可按照调度算法的不同而增删。此调度程序应当包含2种不同的调度算法,运行时可以任选一种,以利于各种方法的分析和比较。而且此程序应能显示或打印各种进程状态和参数变化情况,便于观察。即要显示每个时间片内各进程的情况,并且指出运行进程及就绪和阻塞队列中的内容。
时间: 2024-01-22 07:20:19 浏览: 24
以下是一个简单的基于优先级的进程调度程序的示例代码。它使用了静态分配的 PCB 数组和简单的调度算法。你可以根据自己的需求进行修改和扩展。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESSES 10
typedef enum {
READY,
RUNNING,
FINISHED
} ProcessState;
typedef struct {
int pid;
int priority;
int chip;
int cputime;
int alltime;
ProcessState state;
struct pcb *next;
} PCB;
PCB pcb[MAX_PROCESSES];
PCB *ready_queue = NULL;
PCB *running_process = NULL;
int current_time = 0;
void initialize_processes() {
int i;
for (i = 0; i < MAX_PROCESSES; i++) {
pcb[i].pid = i + 1;
pcb[i].priority = rand() % 10 + 1;
pcb[i].chip = rand() % 5 + 1;
pcb[i].cputime = 0;
pcb[i].alltime = rand() % 10 + 1;
pcb[i].state = READY;
pcb[i].next = NULL;
}
}
void print_processes() {
int i;
printf("PID\tPriority\tChip\tCPU\tAllTime\tState\n");
for (i = 0; i < MAX_PROCESSES; i++) {
printf("%d\t%d\t\t%d\t%d\t%d\t", pcb[i].pid, pcb[i].priority, pcb[i].chip, pcb[i].cputime, pcb[i].alltime);
switch (pcb[i].state) {
case READY:
printf("Ready\n");
break;
case RUNNING:
printf("Running\n");
break;
case FINISHED:
printf("Finished\n");
break;
}
}
}
void add_to_ready_queue(PCB *p) {
if (ready_queue == NULL) {
ready_queue = p;
} else {
PCB *q = ready_queue;
while (q->next != NULL) {
q = q->next;
}
q->next = p;
}
p->state = READY;
}
PCB *get_highest_priority_process() {
PCB *p = ready_queue;
PCB *highest_priority_process = p;
while (p != NULL) {
if (p->priority > highest_priority_process->priority) {
highest_priority_process = p;
}
p = p->next;
}
return highest_priority_process;
}
void schedule() {
PCB *next_process = get_highest_priority_process();
if (running_process != NULL) {
running_process->state = READY;
add_to_ready_queue(running_process);
}
next_process->state = RUNNING;
running_process = next_process;
}
void run_process() {
running_process->cputime++;
running_process->alltime--;
if (running_process->alltime == 0) {
running_process->state = FINISHED;
running_process = NULL;
} else if (running_process->chip == running_process->cputime) {
running_process->state = READY;
add_to_ready_queue(running_process);
running_process = NULL;
}
}
int main() {
int i;
srand(time(NULL));
initialize_processes();
print_processes();
while (1) {
printf("Time %d:\n", current_time);
for (i = 0; i < MAX_PROCESSES; i++) {
if (pcb[i].state == FINISHED) {
continue;
}
if (pcb[i].state == READY) {
printf("Process %d is ready.\n", pcb[i].pid);
}
if (pcb[i].state == RUNNING) {
printf("Process %d is running.\n", pcb[i].pid);
}
}
if (running_process == NULL) {
if (ready_queue == NULL) {
printf("No processes.\n");
break;
} else {
schedule();
}
} else {
run_process();
if (running_process == NULL && ready_queue != NULL) {
schedule();
}
}
current_time++;
}
return 0;
}
```
该程序使用随机生成的进程参数,并使用基于优先级的调度算法。在每个时间片内,程序会输出当前所有进程的状态,以及哪个进程正在运行或等待。当所有进程都完成时,程序会停止并退出。
相关推荐
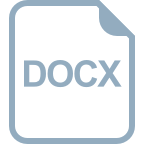
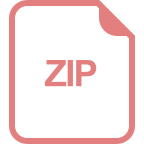
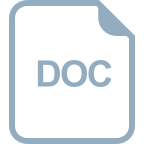















