编写一个圆柱类,该类拥有成员变量,两个构造器方法五个成员方法
时间: 2024-05-07 12:16:43 浏览: 116
好的,我会回答您的问题。以下是编写圆柱类的代码示例:
```java
public class Cylinder {
// 成员变量
private double radius; // 圆柱底面半径
private double height; // 圆柱高
// 构造器方法
public Cylinder() { // 无参构造器
this.radius = 1.0;
this.height = 1.0;
}
public Cylinder(double radius, double height) { // 带参构造器
this.radius = radius;
this.height = height;
}
// 成员方法
public double getRadius() { // 获取圆柱底面半径
return radius;
}
public void setRadius(double radius) { // 设置圆柱底面半径
this.radius = radius;
}
public double getHeight() { // 获取圆柱高
return height;
}
public void setHeight(double height) { // 设置圆柱高
this.height = height;
}
public double getVolume() { // 计算圆柱体积
return Math.PI * radius * radius * height;
}
public double getSurfaceArea() { // 计算圆柱表面积
return 2 * Math.PI * radius * height + 2 * Math.PI * radius * radius;
}
public String toString() { // 将圆柱对象转化为字符串
return "Cylinder [radius=" + radius + ", height=" + height + "]";
}
}
```
其中,成员变量有圆柱底面半径和圆柱高,分别使用私有访问修饰符进行修饰。构造器方法有无参构造器和带参构造器,通过传入参数来初始化成员变量。成员方法有获取和设置圆柱底面半径、圆柱高、计算圆柱体积、计算圆柱表面积、将圆柱对象转化为字符串等。以下是如何使用该类的示例代码:
```java
public class TestCylinder {
public static void main(String[] args) {
Cylinder cylinder1 = new Cylinder(); // 创建一个无参构造器的圆柱对象
Cylinder cylinder2 = new Cylinder(2.0, 5.0); // 创建一个带参构造器的圆柱对象
cylinder1.setRadius(1.5); // 设置圆柱底面半径
cylinder1.setHeight(3.0); // 设置圆柱高
System.out.println(cylinder1.toString()); // 输出圆柱1的信息
System.out.println("圆柱1的体积为:" + cylinder1.getVolume()); // 输出圆柱1的体积
System.out.println("圆柱1的表面积为:" + cylinder1.getSurfaceArea()); // 输出圆柱1的表面积
System.out.println(cylinder2.toString()); // 输出圆柱2的信息
System.out.println("圆柱2的体积为:" + cylinder2.getVolume()); // 输出圆柱2的体积
System.out.println("圆柱2的表面积为:" + cylinder2.getSurfaceArea()); // 输出圆柱2的表面积
}
}
```
以上就是编写圆柱类的过程,同时也回答了您的问题。希望对您有所帮助!
阅读全文
相关推荐
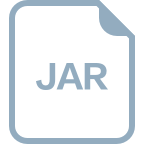















