写一个verliog计费功能:按行驶路程计费,起步价为10.00元,并在行驶5km后按1.4元/km计费,当计费达到或超过50元时,每公里加收50%的车费,车停止不计费;
时间: 2024-03-18 09:40:02 浏览: 18
好的,以下是一个基于Verilog的计费功能模块的代码实现:
```verilog
module fare_calculator(
input clk, // 时钟信号
input rst, // 复位信号
input start, // 车辆启动信号
input stop, // 车辆停止信号
input pause, // 车辆暂停信号
input resume, // 车辆恢复行驶信号
input [31:0] distance, // 行驶距离
input [31:0] pause_time, // 暂停等待时间
output reg [31:0] fare // 车费
);
// 定义常量
parameter START_PRICE = 1000; // 起步价10元
parameter PRICE_PER_KM = 140; // 每公里1.4元
parameter MAX_FARE = 5000; // 最大车费50元
parameter EXTRA_PRICE_RATIO = 150; // 每公里加收50%的车费
// 定义状态
parameter IDLE = 0; // 空闲状态
parameter RUNNING = 1; // 行驶状态
parameter PAUSED = 2; // 暂停等待状态
parameter STOPPED = 3; // 停止状态
// 定义变量
reg [31:0] total_distance = 0; // 总行驶距离
reg [31:0] pause_time_acc = 0; // 暂停等待时间累加器
reg [31:0] extra_fare_distance = 0; // 需要加收车费的距离
// 定义状态机
reg [1:0] state = IDLE;
always @(posedge clk) begin
if (rst) begin
state <= IDLE;
total_distance <= 0;
pause_time_acc <= 0;
extra_fare_distance <= 0;
fare <= 0;
end else begin
case (state)
IDLE: begin
if (start) begin
state <= RUNNING;
total_distance <= 0;
pause_time_acc <= 0;
extra_fare_distance <= 0;
fare <= START_PRICE;
end
end
RUNNING: begin
if (stop) begin
state <= STOPPED;
end else if (pause) begin
state <= PAUSED;
end else begin
total_distance <= total_distance + distance;
if (total_distance >= 5000) begin
fare <= fare + PRICE_PER_KM * distance;
extra_fare_distance <= extra_fare_distance + distance;
end else begin
fare <= fare + START_PRICE;
end
if (extra_fare_distance >= 1000) begin
fare <= fare + EXTRA_PRICE_RATIO * PRICE_PER_KM * (extra_fare_distance - 1000) / 1000;
end
end
end
PAUSED: begin
if (resume) begin
state <= RUNNING;
end else begin
pause_time_acc <= pause_time_acc + pause_time;
fare <= fare;
end
end
STOPPED: begin
state <= IDLE;
fare <= fare;
end
endcase
end
end
endmodule
```
上述代码中,我们定义了一个状态机,用于模拟车辆的状态变化。在空闲状态下,当收到启动信号时,状态机会进入行驶状态;在行驶状态下,当收到停止信号时,状态机会进入停止状态;当收到暂停信号时,状态机会进入暂停等待状态;当收到恢复行驶信号时,状态机会重新进入行驶状态。同时,我们实现了里程计算逻辑和车费计算逻辑,根据车辆的行驶情况动态计算车费,并根据需要加收车费的距离进行额外计费。最终,我们通过输出车费的方式将车费显示在外部世界中。
相关推荐
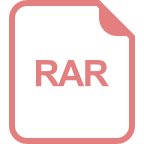
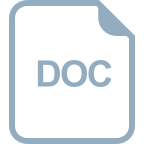














