用uniapp写一个糖豆人游戏
时间: 2023-05-20 10:01:31 浏览: 91
非常感谢您的提问!以下是一个简单的糖豆人游戏的示例代码,使用uniapp框架编写:
<template>
<div class="container">
<div class="game-board">
<div class="row" v-for="(row, rowIndex) in board" :key="rowIndex">
<div class="cell" v-for="(cell, colIndex) in row" :key="colIndex" @click="handleClick(rowIndex, colIndex)">
<img :src="getImage(cell)" />
</div>
</div>
</div>
<div class="score">得分: {{ score }}</div>
</div>
</template>
<script>
export default {
data() {
return {
board: [], // 游戏棋盘
score: 0, // 得分
selected: null, // 当前选中的糖豆人
};
},
mounted() {
this.initBoard();
},
methods: {
// 初始化游戏棋盘
initBoard() {
const colors = ['red', 'yellow', 'green', 'blue', 'purple'];
const board = [];
for (let i = 0; i < 8; i++) {
const row = [];
for (let j = 0; j < 8; j++) {
const color = colors[Math.floor(Math.random() * colors.length)];
row.push({
color,
selected: false,
});
}
board.push(row);
}
this.board = board;
},
// 处理点击事件
handleClick(rowIndex, colIndex) {
const cell = this.board[rowIndex][colIndex];
if (cell.color === 'gray') {
return;
}
if (this.selected) {
if (this.isAdjacent(rowIndex, colIndex)) {
this.swapCells(rowIndex, colIndex);
const matches = this.findMatches();
if (matches.length > 0) {
this.removeMatches(matches);
this.fillBoard();
this.score += matches.length;
} else {
this.swapCells(rowIndex, colIndex);
this.swapCells(this.selected.rowIndex, this.selected.colIndex);
}
this.selected = null;
} else {
this.selected.selected = false;
cell.selected = true;
this.selected = {
rowIndex,
colIndex,
};
}
} else {
cell.selected = true;
this.selected = {
rowIndex,
colIndex,
};
}
},
// 判断两个格子是否相邻
isAdjacent(rowIndex, colIndex) {
const { rowIndex: selectedRowIndex, colIndex: selectedColIndex } = this.selected;
return (
(rowIndex === selectedRowIndex && Math.abs(colIndex - selectedColIndex) === 1) ||
(colIndex === selectedColIndex && Math.abs(rowIndex - selectedRowIndex) === 1)
);
},
// 交换两个格子
swapCells(rowIndex, colIndex) {
const { rowIndex: selectedRowIndex, colIndex: selectedColIndex } = this.selected;
const temp = this.board[rowIndex][colIndex];
this.board[rowIndex][colIndex] = this.board[selectedRowIndex][selectedColIndex];
this.board[selectedRowIndex][selectedColIndex] = temp;
},
// 查找所有匹配的糖豆人
findMatches() {
const matches = [];
for (let i = 0; i < 8; i++) {
for (let j = 0; j < 8; j++) {
const cell = this.board[i][j];
if (cell.color !== 'gray') {
const horizontalMatches = this.findHorizontalMatches(i, j);
const verticalMatches = this.findVerticalMatches(i, j);
if (horizontalMatches.length >= 3) {
matches.push(...horizontalMatches);
}
if (verticalMatches.length >= 3) {
matches.push(...verticalMatches);
}
}
}
}
return matches;
},
// 查找水平方向上的匹配
findHorizontalMatches(rowIndex, colIndex) {
const matches = [];
const color = this.board[rowIndex][colIndex].color;
let left = colIndex - 1;
while (left >= 0 && this.board[rowIndex][left].color === color) {
matches.push({
rowIndex,
colIndex: left,
});
left--;
}
let right = colIndex + 1;
while (right < 8 && this.board[rowIndex][right].color === color) {
matches.push({
rowIndex,
colIndex: right,
});
right++;
}
matches.push({
rowIndex,
colIndex,
});
return matches;
},
// 查找垂直方向上的匹配
findVerticalMatches(rowIndex, colIndex) {
const matches = [];
const color = this.board[rowIndex][colIndex].color;
let top = rowIndex - 1;
while (top >= 0 && this.board[top][colIndex].color === color) {
matches.push({
rowIndex: top,
colIndex,
});
top--;
}
let bottom = rowIndex + 1;
while (bottom < 8 && this.board[bottom][colIndex].color === color) {
matches.push({
rowIndex: bottom,
colIndex,
});
bottom++;
}
matches.push({
rowIndex,
colIndex,
});
return matches;
},
// 移除所有匹配的糖豆人
removeMatches(matches) {
matches.forEach(({ rowIndex, colIndex }) => {
this.board[rowIndex][colIndex].color = 'gray';
});
},
// 填充空缺的格子
fillBoard() {
for (let j = 0; j < 8; j++) {
let emptyCount = 0;
for (let i = 7; i >= 0; i--) {
const cell = this.board[i][j];
if (cell.color === 'gray') {
emptyCount++;
} else if (emptyCount > 0) {
this.board[i + emptyCount][j] = cell;
this.board[i][j] = {
color: 'gray',
selected: false,
};
}
}
for (let i = 0; i < emptyCount; i++) {
const color = ['red', 'yellow', 'green', 'blue', 'purple'][Math.floor(Math.random() * 5)];
this.board[i][j] = {
color,
selected: false,
};
}
}
},
// 获取糖豆人的图片路径
getImage(cell) {
return `./images/${cell.color}.png`;
},
},
};
</script>
<style>
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
}
.game-board {
display: flex;
flex-wrap: wrap;
width: 320px;
height: 320px;
border: 1px solid #ccc;
}
.row {
display: flex;
flex-wrap: wrap;
width: 100%;
}
.cell {
display: flex;
align-items: center;
justify-content: center;
width: 37.5px;
height: 37.5px;
cursor: pointer;
}
.cell img {
width: 30px;
height: 30px;
}
.score {
margin-top: 20px;
font-size: 24px;
font-weight: bold;
}
</style>
注意:以上代码仅供参考,可能存在错误或不完善的地方,欢迎指正。
相关推荐
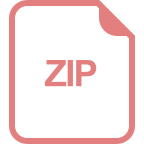
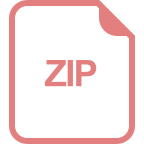














