对文件夹中数据集扩充代码
时间: 2023-08-06 14:03:21 浏览: 165
对文件夹中的数据集进行增强可以使用Python中的OS和Pillow库。以下是一个对文件夹中的图像进行随机旋转和水平翻转的示例代码:
```python
import os
import random
from PIL import Image
# 数据集路径
dataset_path = "dataset/"
# 旋转角度范围
rotate_range = (-30, 30)
# 随机旋转图像
def random_rotate(image):
# 随机旋转角度
angle = random.randint(rotate_range[0], rotate_range[1])
# 旋转图像
return image.rotate(angle)
# 随机水平翻转图像
def random_flip(image):
# 随机水平翻转
if random.random() < 0.5:
return image.transpose(method=Image.FLIP_LEFT_RIGHT)
else:
return image
# 对文件夹中的图像进行增强
def augment_dataset(dataset_path):
for root, dirs, files in os.walk(dataset_path):
for file in files:
if file.endswith(".jpg") or file.endswith(".png"):
# 读取图像
image_path = os.path.join(root, file)
image = Image.open(image_path)
# 随机旋转和水平翻转
image = random_rotate(image)
image = random_flip(image)
# 保存增强后的图像
new_image_path = os.path.join(root, "aug_" + file)
image.save(new_image_path)
augment_dataset(dataset_path)
```
以上代码会遍历指定路径下的所有文件夹和图像文件,并对每个图像进行随机旋转和水平翻转,然后保存增强后的图像文件,文件名以"aug_"前缀区分。可以根据实际需求,修改增强方式和参数,例如添加噪声、缩放、裁剪等操作。
阅读全文
相关推荐
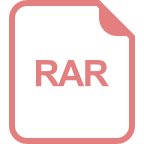
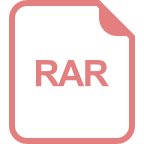
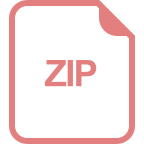











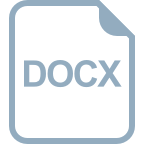