c++sort函数怎么用
时间: 2024-09-07 13:03:43 浏览: 43
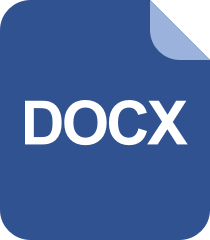
c++ sort函数使用总结
在C++中,`sort` 函数用于对序列进行排序,其原型定义在 `<algorithm>` 头文件中。最常用的版本是 `std::sort`,其基本用法如下:
```cpp
#include <algorithm> // 包含sort函数的头文件
#include <vector> // 包含vector容器的头文件,排序通常用于容器
int main() {
// 假设有一个整数数组
int arr[] = {5, 2, 4, 6, 1, 3};
// 计算数组的元素个数
size_t n = sizeof(arr) / sizeof(*arr);
// 使用sort函数进行排序
std::sort(arr, arr + n); // 默认升序排序
// ... 其他代码 ...
return 0;
}
```
如果你想要对自定义类型的数组或者其他容器进行排序,你可能需要提供一个比较函数或者重载 `operator<`。例如:
```cpp
#include <algorithm>
#include <vector>
#include <iostream>
// 自定义类型
struct MyType {
int value;
// 其他成员变量
// 如果想要使用默认的升序排序,需要重载<运算符
bool operator<(const MyType& other) const {
return value < other.value;
}
};
int main() {
// 使用自定义类型的向量
std::vector<MyType> vec;
vec.push_back({3});
vec.push_back({1});
vec.push_back({2});
// 使用sort对自定义类型的向量进行排序
std::sort(vec.begin(), vec.end());
// 输出排序结果
for (const auto& item : vec) {
std::cout << item.value << " ";
}
std::cout << std::endl;
// ... 其他代码 ...
return 0;
}
```
`std::sort` 函数接受两个迭代器参数,它们定义了要排序的序列的范围。默认情况下,`std::sort` 使用小于运算符(`<`)来比较元素。如果你希望以其他方式排序,比如降序,你可以传递一个比较函数。
以下是一个降序排序的例子:
```cpp
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {5, 2, 4, 6, 1, 3};
// 使用比较函数进行降序排序
std::sort(vec.begin(), vec.end(), std::greater<int>());
// 输出排序结果
for (int num : vec) {
std::cout << num << " ";
}
std::cout << std::endl;
// ... 其他代码 ...
return 0;
}
```
这里使用了 `std::greater<int>()` 作为第三个参数,它是一个函数对象,用于实现降序排序。
阅读全文
相关推荐
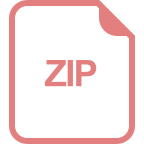
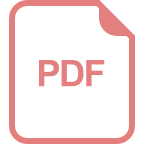










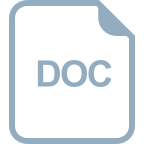




