写一个雷霆战机c语言程序
时间: 2024-06-08 10:09:09 浏览: 15
以下是一个简单的雷霆战机游戏的C语言程序,仅供参考:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 50
#define HEIGHT 25
#define PLANE_WIDTH 5
#define PLANE_HEIGHT 3
void gotoxy(int x, int y);
void draw_plane(int x, int y);
void draw_enemy(int x, int y);
void clear_bullet(int x, int y);
void draw_bullet(int x, int y);
void clear_enemy(int x, int y);
void move_enemy(int *x, int *y);
void move_bullet(int *x, int *y, int *state);
bool is_hit(int x1, int y1, int x2, int y2);
void game_over();
int main() {
int score = 0;
int plane_x = WIDTH / 2 - PLANE_WIDTH / 2;
int plane_y = HEIGHT - 3;
int enemy_x = rand() % (WIDTH - 2) + 1;
int enemy_y = 1;
bool is_game_over = false;
// 隐藏光标
CONSOLE_CURSOR_INFO cursor_info = {1, 0};
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
// 绘制边框
for (int i = 0; i < WIDTH; i++) {
gotoxy(i, 0);
printf("#");
gotoxy(i, HEIGHT - 1);
printf("#");
}
for (int i = 1; i < HEIGHT - 1; i++) {
gotoxy(0, i);
printf("#");
gotoxy(WIDTH - 1, i);
printf("#");
}
// 绘制飞机
draw_plane(plane_x, plane_y);
while (!is_game_over) {
// 移动敌机
move_enemy(&enemy_x, &enemy_y);
// 绘制敌机
draw_enemy(enemy_x, enemy_y);
// 发射子弹
if (kbhit()) {
int key = getch();
if (key == ' ') {
int bullet_x = plane_x + PLANE_WIDTH / 2;
int bullet_y = plane_y - 1;
int bullet_state = 1;
draw_bullet(bullet_x, bullet_y);
while (bullet_y > 0) {
clear_bullet(bullet_x, bullet_y);
move_bullet(&bullet_x, &bullet_y, &bullet_state);
draw_bullet(bullet_x, bullet_y);
// 判断是否击中敌机
if (is_hit(bullet_x, bullet_y, enemy_x, enemy_y)) {
score++;
gotoxy(WIDTH + 2, 2);
printf("Score: %d", score);
clear_enemy(enemy_x, enemy_y);
enemy_x = rand() % (WIDTH - 2) + 1;
enemy_y = 1;
break;
}
}
}
}
// 判断是否被撞击
if (is_hit(plane_x, plane_y, enemy_x, enemy_y)) {
is_game_over = true;
}
Sleep(50);
}
game_over();
return 0;
}
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_plane(int x, int y) {
gotoxy(x, y);
printf(" %c ", 219);
gotoxy(x, y + 1);
printf(" %c%c%c ", 219, 219, 219);
gotoxy(x, y + 2);
printf("%c %c %c", 219, 219, 219);
}
void draw_enemy(int x, int y) {
gotoxy(x, y);
printf("%c%c%c", 30, 40, 30);
gotoxy(x, y + 1);
printf("%c%c%c", 94, 88, 94);
gotoxy(x, y + 2);
printf("%c%c%c", 94, 88, 94);
}
void clear_bullet(int x, int y) {
gotoxy(x, y);
printf(" ");
}
void draw_bullet(int x, int y) {
gotoxy(x, y);
printf("|");
}
void clear_enemy(int x, int y) {
gotoxy(x, y);
printf(" ");
gotoxy(x, y + 1);
printf(" ");
gotoxy(x, y + 2);
printf(" ");
}
void move_enemy(int *x, int *y) {
clear_enemy(*x, *y);
(*y)++;
if (*y >= HEIGHT - 1) {
*x = rand() % (WIDTH - 2) + 1;
*y = 1;
}
}
void move_bullet(int *x, int *y, int *state) {
if (*state == 1) {
(*y)--;
if (*y <= 0) {
*state = 0;
}
}
}
bool is_hit(int x1, int y1, int x2, int y2) {
return (x1 >= x2 && x1 <= x2 + 2 && y1 >= y2 && y1 <= y2 + 2);
}
void game_over() {
system("cls");
gotoxy(WIDTH / 2 - 5, HEIGHT / 2);
printf("Game Over!");
getch();
}
```
相关推荐
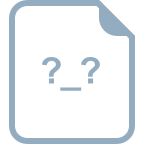














