std::string& name作用
时间: 2024-06-22 12:02:54 浏览: 5
`std::string& name` 是 C++ 中的一个引用类型,它代表一个 `std::string` 类型的引用。在这个上下文中,`name` 可能是一个变量名,用来引用一个已存在的字符串对象。使用引用的原因有:
1. **效率提升**:引用比复制对象更高效,因为它们不涉及实际的数据拷贝,直接操作的是原始对象的内存地址。
2. **避免临时对象**:如果你在函数参数中传递一个字符串,用 `std::string&` 可以避免创建临时副本,尤其是在处理大量数据时。
3. **改变被引用的对象**:引用可以直接修改它所指向的对象,这在需要修改字符串内容的函数中很常见。
相关问题:
1. 什么是指针和引用的区别?
2. 引用在哪些情况下会用到 const 追加,例如 `std::string const&`?
3. C++ 中如何声明和初始化一个引用?
相关问题
std::string &name
std::string &name是一个引用类型的变量,它引用了一个std::string类型的对象。通过引用,我们可以直接操作原始对象,而不需要进行拷贝。下面是两种使用引用遍历std::string对象的例子:
1. 使用const_iterator遍历std::string对象:
```cpp
for(std::string::const_iterator cit = name.begin(); cit != name.end(); ++cit) {
// 对每个字符执行操作
}
```
2. 使用iterator遍历std::string对象:
```cpp
for(std::string::iterator it = name.begin(); it != name.end(); ++it) {
// 对每个字符执行操作
}
```
这两种遍历方式的区别在于const_iterator是一个只读迭代器,而iterator是一个可读写的迭代器。如果你只需要读取std::string对象的字符,可以使用const_iterator;如果你需要修改std::string对象的字符,可以使用iterator。
如何实现std::string getUniqueName(const std::string&name, std::vector<std::string>& names, int d );,请用c++实现
以下是一个可能的实现:
```cpp
#include <string>
#include <vector>
#include <unordered_set>
std::string getUniqueName(const std::string& name, std::vector<std::string>& names, int d)
{
std::unordered_set<std::string> usedNames;
for (const auto& n : names) {
usedNames.insert(n);
}
std::string uniqueName = name;
for (int i = 0; i < d; ++i) {
if (usedNames.find(uniqueName) == usedNames.end()) {
names.push_back(uniqueName);
return uniqueName;
}
uniqueName = name + std::to_string(i);
}
names.push_back(uniqueName);
return uniqueName;
}
```
这个函数会在 `names` 中查找是否已经存在名字为 `name` 或以 `name` 为前缀加数字后缀的字符串,如果不存在就返回 `name` 或者以 `name` 为前缀加数字后缀的字符串,并将新名字保存到 `names` 中。`d` 参数表示数字后缀的最大值,例如 `d=3` 表示数字后缀最大为 3。如果所有可能的名字都已经被占用,函数会返回以 `name` 为前缀加数字后缀的字符串,并将新名字保存到 `names` 中。
相关推荐
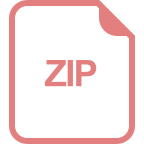
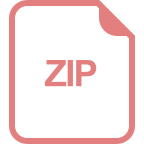
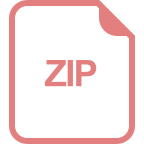












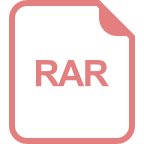