抽烟者问题linux代码
时间: 2023-10-18 14:02:59 浏览: 93
抽烟者问题是一个经典的并发编程问题,描述了三个进程(抽烟者进程和一个中介进程)之间的竞争关系。
抽烟者问题的场景是:有三个抽烟者,每个抽烟者只有部分材料(烟草、纸和胶水)可以用来制作香烟。同时有一个中介进程,负责提供这三种材料中的两种给抽烟者。但是同一时间只能有一个抽烟者在制作香烟,其他两个则等待。如果某个抽烟者缺少某种材料,他就无法制作香烟。
以下是一个基于Linux环境的解决方案:
```C++
#include <stdio.h>
#include <pthread.h>
#include <semaphore.h>
sem_t tobacco_sem, paper_sem, glue_sem, agent_sem;
void *agent(void *arg) {
while (1) {
sem_wait(&agent_sem);
int r = rand() % 3;
if (r == 0) {
sem_post(&tobacco_sem);
sem_post(&paper_sem);
} else if (r == 1) {
sem_post(&tobacco_sem);
sem_post(&glue_sem);
} else {
sem_post(&paper_sem);
sem_post(&glue_sem);
}
}
}
void *smoker_tobacco(void *arg) {
while (1) {
sem_wait(&tobacco_sem);
sem_wait(&paper_sem);
printf("Smoker with tobacco is making a cigarette.\n");
sem_post(&agent_sem);
}
}
void *smoker_paper(void *arg) {
while (1) {
sem_wait(&paper_sem);
sem_wait(&glue_sem);
printf("Smoker with paper is making a cigarette.\n");
sem_post(&agent_sem);
}
}
void *smoker_glue(void *arg) {
while (1) {
sem_wait(&tobacco_sem);
sem_wait(&glue_sem);
printf("Smoker with glue is making a cigarette.\n");
sem_post(&agent_sem);
}
}
int main() {
srand(time(NULL));
sem_init(&tobacco_sem, 0, 0);
sem_init(&paper_sem, 0, 0);
sem_init(&glue_sem, 0, 0);
sem_init(&agent_sem, 0, 1);
pthread_t agent_thread, smoker_tobacco_thread, smoker_paper_thread, smoker_glue_thread;
pthread_create(&agent_thread, NULL, agent, NULL);
pthread_create(&smoker_tobacco_thread, NULL, smoker_tobacco, NULL);
pthread_create(&smoker_paper_thread, NULL, smoker_paper, NULL);
pthread_create(&smoker_glue_thread, NULL, smoker_glue, NULL);
pthread_join(agent_thread, NULL);
pthread_join(smoker_tobacco_thread, NULL);
pthread_join(smoker_paper_thread, NULL);
pthread_join(smoker_glue_thread, NULL);
sem_destroy(&tobacco_sem);
sem_destroy(&paper_sem);
sem_destroy(&glue_sem);
sem_destroy(&agent_sem);
return 0;
}
```
以上是一个简化的解决方案,通过`pthread`库创建了代表中介进程和抽烟者进程的线程,并使用信号量`semaphore`来控制资源的访问。其中`agent`线程负责提供材料,`smoker_tobacco`、`smoker_paper`和`smoker_glue`线程分别代表三种抽烟者,它们根据拥有的材料情况来制作香烟。
阅读全文
相关推荐
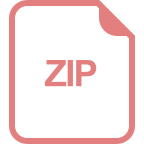
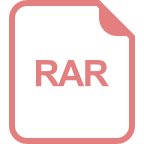
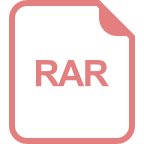















