.写顺序表插入操作的自定义函数,在顺序表第i个位置插入元素(i的值和插入元素的值可自由更改,注意非法插入问题,并进行顺序表的判满操作),在主函数内调用此函数,并打印输出各元素查看结果
时间: 2024-09-30 14:12:34 浏览: 36
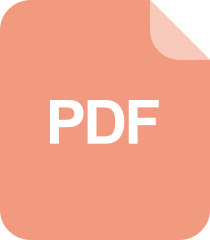
C++顺序表的基本操作(使用模版类)

在C++中,你可以创建一个顺序表(数组实现)并为其提供一个自定义函数`insertAtPosition`来完成插入操作。首先,你需要定义一个节点结构体和一个顺序表容器,这里假设你已经有一个模板类`SequentialList`作为基础。
```cpp
template <typename T>
class SequentialListNode {
public:
T data;
SequentialListNode<T>* next;
// 构造函数
SequentialListNode(T value) : data(value), next(nullptr) {}
};
template <typename T>
class SequentialList {
private:
int size;
SequentialListNode<T>* head;
public:
SequentialList() : size(0), head(nullptr) {}
// 插入函数,检查索引合法性
void insertAtPosition(int i, T value) {
if (i < 0 || i > size) {
throw std::out_of_range("Invalid index");
}
// 如果列表已满,先删除最后一个元素腾出空间
if (size == capacity()) {
resize(capacity() * 2); // 预估更多空间
}
// 创建新节点
SequentialListNode<T>* newNode = new SequentialListNode<T>(value);
if (i == 0) { // 插入到头部
newNode->next = head;
head = newNode;
} else {
SequentialListNode<T>* current = head;
for (int j = 0; j < i - 1; ++j) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
size++;
}
// 判断列表是否已满,这里假设每次扩容两倍
bool isFull() const {
return size >= capacity();
}
// 打印顺序表内容
void printList() const {
SequentialListNode<T>* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
private:
// 调整容量大小(实际实现中可能需要处理边界情况)
void resize(int newCapacity) {
delete[] head; // 释放旧空间
head = new SequentialListNode<T>[newCapacity];
size = 0;
}
// 容量大小(这里设为最大元素数,可以根据实际情况调整)
static constexpr int capacity() { return 10; }
};
```
然后,在主函数`main`中,你可以创建一个`SequentialList<int>`实例,调用`insertAtPosition`并打印列表:
```cpp
int main() {
SequentialList<int> list;
list.insertAtPosition(0, 5);
list.insertAtPosition(2, 10); // 插入5和10
try {
list.insertAtPosition(-1, 15); // 非法插入
} catch (const std::out_of_range& e) {
std::cerr << "Error: " << e.what() << '\n';
}
list.printList();
return 0;
}
```
运行这个程序,你会看到合法插入后的顺序表和插入异常的错误信息。
阅读全文
相关推荐
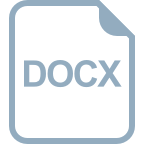
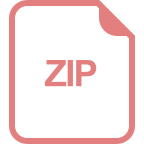















