DHT11驱动代码,STM32F103ZET6
时间: 2023-08-31 11:26:26 浏览: 130
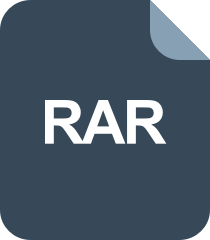
DHT11与STM32F103ZET6

### 回答1:
以下是DHT11驱动代码,适用于STM32F103ZET6单片机。
```c
#include "stm32f10x.h"
#include "dht11.h"
#include <stdio.h>
// 定义DHT11的引脚
#define DHT11_PORT GPIOB
#define DHT11_PIN GPIO_Pin_0
// 延时函数
void Delay_us(uint32_t us)
{
uint32_t i;
for(i = 0; i < us; i++)
{
__nop();
__nop();
}
}
// 初始化DHT11引脚
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
}
// 发送起始信号
void DHT11_Start(void)
{
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_us(1000);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
Delay_us(18000);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_us(40);
}
// 接收DHT11的响应
uint8_t DHT11_Response(void)
{
uint8_t retry = 0;
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) // 等待DHT11将总线拉低
{
retry++;
Delay_us(1);
}
if(retry >= 100) // 如果总线一直为高电平,则无响应
return 1;
else
retry = 0;
while(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) && retry < 100) // 等待DHT11将总线拉高
{
retry++;
Delay_us(1);
}
if(retry >= 100) // 如果总线一直为低电平,则无响应
return 1;
else
return 0;
}
// 读取一个字节的数据
uint8_t DHT11_ReadByte(void)
{
uint8_t i, data = 0;
for(i = 0; i < 8; i++)
{
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == 0); // 等待DHT11将总线拉高,表示数据位开始
Delay_us(40);
if(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == 1) // 如果数据位为1
data |= (1 << (7 - i)); // 将第i位设为1
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待DHT11将总线拉低,表示数据位结束
}
return data;
}
// 读取DHT11的温湿度数据
uint8_t DHT11_ReadData(uint8_t *temp, uint8_t *humi)
{
uint8_t i, buf[5];
DHT11_Start(); // 发送起始信号
if(DHT11_Response() == 1) // 接收响应
return 1;
for(i = 0; i < 5; i++) // 读取40位数据
buf[i] = DHT11_ReadByte();
if((uint8_t)(buf[0] + buf[1] + buf[2] + buf[3]) == buf[4]) // 校验数据
{
*humi = buf[0];
*temp = buf[2];
return 0;
}
else
return 1;
}
int main(void)
{
uint8_t temp, humi;
DHT11_Init();
while(1)
{
if(DHT11_ReadData(&temp, &humi) == 0)
printf("Temperature: %dC, Humidity: %d%%\n", temp, humi);
else
printf("Failed to read data from DHT11.\n");
Delay_us(1000000);
}
}
```
### 回答2:
DHT11是一种数字温湿度传感器,可通过STM32F103ZET6微控制器进行驱动。以下是一个简单的DHT11驱动代码示例:
1. 首先,配置引脚,将DHT11的数据引脚连接到STM32的一个GPIO引脚上,并设置该引脚为输入模式。
```c
#define DHT11_PIN GPIO_Pin_0
#define DHT11_PORT GPIOA
...
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = DHT11_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStruct);
```
2. 为了从DHT11读取数据,我们需要发送一个开始信号,通过GPIO引脚将其置为低电平,然后延时一段时间再将其置为高电平。
```c
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
Delay_Microsecond(18000);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_Microsecond(20);
```
3. 等待DHT11的响应,DHT11会将引脚拉低一段时间作为响应信号。
```c
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_SET);
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_RESET);
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_SET);
```
4. 开始接收温湿度数据,DHT11会按位发送数据,通过对引脚的电平进行测量来解码数据。
```c
uint8_t data[5];
for(uint8_t i=0; i<5; i++){
for(uint8_t j=0; j<8; j++){
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_RESET);
Delay_Microsecond(40);
if(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_SET){
data[i] |= (1 << (7-j));
}
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == Bit_SET);
}
}
```
5. 最后,保存收到的数据,并计算温度和湿度值。
```c
uint8_t humidity = data[0];
uint8_t temperature = data[2];
```
以上是一个简单的DHT11驱动代码示例,用于STM32F103ZET6微控制器读取DHT11传感器的温湿度数据。实际代码可能需要根据硬件连接和其他功能做适当的调整和优化。
### 回答3:
DHT11是一款数字温湿度传感器,可以与STM32F103ZET6单片机进行连接。下面是一个简单的DHT11驱动代码示例:
```c
#include "stm32f10x.h"
#define DHT11_PIN GPIO_Pin_0
#define DHT11_PORT GPIOB
void DHT11_Init()
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
delay_ms(18);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
delay_us(20);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
}
uint8_t DHT11_ReadByte()
{
uint8_t byte = 0;
uint8_t i;
for(i = 0; i < 8; i++)
{
while(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待高电平
delay_us(30);
if(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
byte = (byte << 1) | 0x01;
else byte <<= 1;
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待低电平
}
return byte;
}
void DHT11_ReadData(uint8_t* temperature, uint8_t* humidity)
{
DHT11_Init();
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待高电平
while(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待低电平
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN)); // 等待高电平
*humidity = DHT11_ReadByte();
*temperature = DHT11_ReadByte();
uint8_t checksum = DHT11_ReadByte();
if(checksum != (*humidity + *temperature))
{
// 数据校验错误
}
}
```
上述代码中,DHT11_Init()函数用于初始化DHT11传感器的连接引脚,DHT11_ReadByte()函数用于读取一个字节的数据,DHT11_ReadData()函数用于读取温湿度数据并进行数据校验。代码中使用了延时函数delay_ms()和delay_us(),需要根据实际情况自行添加或替换为相应的延时函数。
请注意,上述代码仅供参考,具体的接线和其他配置可能需要根据具体的STM32F103ZET6开发板和DHT11传感器的引脚定义来修改。
阅读全文
相关推荐
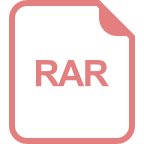
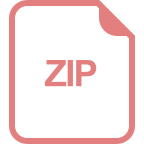


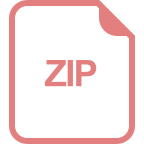
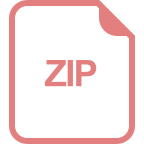
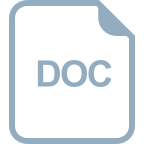
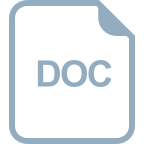
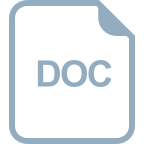
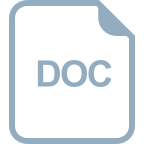
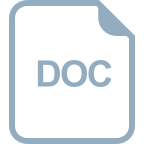
